This tutorial explains how to use MOXy JSON feature with Jersey 2.x. MOXy is the default JSON-Binding Provider in Jersey 2.x. Though I still personally prefer Jackson over MOXy for performance reasons.
Table of Contents MOXy maven dependencies/changes REST API code Model bean changes Manually adding MoxyJsonFeature Customize behavior using MoxyJsonConfig Demo
MOXy maven dependencies/changes
MOXy media module is one of the modules in Jersey 2.x where you don’t need to explicitly register it’s features e.g. MoxyJsonFeature
. Once Jersey detects added it’s presence in class path, it automatically registers it. So just add MOXy dependency in pom.xml
does half work.
<dependency> <groupId>org.glassfish.jersey.media</groupId> <artifactId>jersey-media-moxy</artifactId> <version>2.19</version> </dependency>
REST API code
At Service side, where your APIs are written, you need to enable JSON media types using @Produces(MediaType.APPLICATION_JSON)
annotation.
JerseyService.java
@Path("/employees") public class JerseyService { @GET @Produces(MediaType.APPLICATION_JSON) public Employees getAllEmployees() { Employees list = new Employees(); list.setEmployeeList(new ArrayList<Employee>()); list.getEmployeeList().add(new Employee(1, "Lokesh Gupta")); list.getEmployeeList().add(new Employee(2, "Alex Kolenchiskey")); list.getEmployeeList().add(new Employee(3, "David Kameron")); return list; } }
Model bean changes
At model beans side, you don’t need to put any annotation or any configuration. It will work by default. You even don’t need to put any root annotation as well.
Employees.java
public class Employees { private List<Employee> employeeList; public List<Employee> getEmployeeList() { return employeeList; } public void setEmployeeList(List<Employee> employeeList) { this.employeeList = employeeList; } }
Employee.java
public class Employee { private Integer id; private String name; public Employee() { } public Employee(Integer id, String name) { this.id = id; this.name = name; } public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } @Override public String toString() { return "Employee [id=" + id + ", name=" + name + "]"; } }
Manually adding MoxyJsonFeature
Though MoxyJsonFeature
is registered automatically, if you wish to register it manually, you can add it in configuration as below.
public class CustomApplication extends Application { //Add Service APIs @Override public Set<Class<?>> getClasses() { Set<Class<?>> resources = new HashSet<Class<?>>(); //register REST modules resources.add(JerseyService.class); //Manually adding MOXyJSONFeature resources.add(org.glassfish.jersey.moxy.json.MoxyJsonFeature.class); //Configure Moxy behavior resources.add(JsonMoxyConfigurationContextResolver.class); return resources; } }
And add this Application class in web.xml
file.
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>jersey-serlvet</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>javax.ws.rs.Application</param-name> <param-value>com.howtodoinjava.jersey.CustomApplication</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>jersey-serlvet</servlet-name> <url-pattern>/rest/*</url-pattern> </servlet-mapping> </web-app>
Customize behavior using MoxyJsonConfig
There are certain features with MOXy provides on top of JAXB, you can enable/disable these features by providing MoxyJsonConfig
implementation.
//Register ContextResolver<MoxyJsonConfig> to override //default behavior of marshaling/un-marshaling attributes @Provider public class JsonMoxyConfigurationContextResolver implements ContextResolver<MoxyJsonConfig> { private final MoxyJsonConfig config; public JsonMoxyConfigurationContextResolver() { final Map<String, String> namespacePrefixMapper = new HashMap<String, String>(); namespacePrefixMapper.put("http://www.w3.org/2001/XMLSchema-instance", "xsi"); config = new MoxyJsonConfig() .setNamespacePrefixMapper(namespacePrefixMapper) .setNamespaceSeparator(':') .setAttributePrefix("") .setValueWrapper("value") .property(JAXBContextProperties.JSON_WRAPPER_AS_ARRAY_NAME, true) .setFormattedOutput(true) .setIncludeRoot(true) .setMarshalEmptyCollections(true); } @Override public MoxyJsonConfig getContext(Class<?> objectType) { return config; } }
JsonMoxyConfigurationContextResolver
has been added in CustomApplication class above at line no. 16.
Demo
Simply deploy your application in any server and hit the URL: http://localhost:8080/JerseyDemos/rest/employees
And this will produce below output.
{ "employeeList": [ { "id": 1, "name": "Lokesh Gupta" }, { "id": 2, "name": "Alex Kolenchiskey" }, { "id": 3, "name": "David Kameron" } ] }
Below is the hierarchy of above files in demo application.
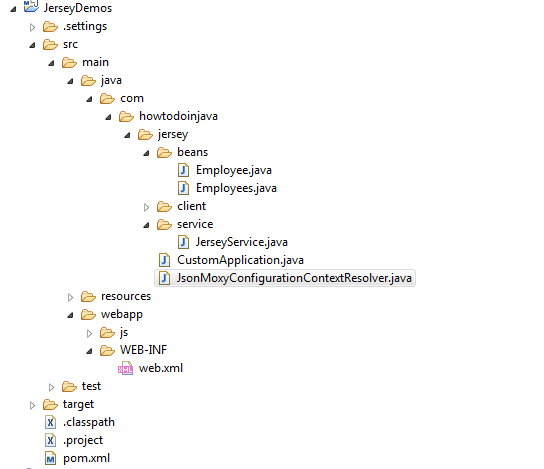
Drop me your question and comments below.
Happy Learning !!
Reference : https://jersey.java.net/documentation/latest/media.html#json.moxy
Comments