In object-oriented languages, the classes are the building blocks of any application. If these blocks are not strong, the building (i.e. the application) will face a tough time in the future. Poorly designed applications can lead the team to very difficult situations when the application scope goes up, or the implementation faces certain design issues either in production or maintenance.
On the other hand, a set of well-designed and written classes can speed up the coding process, while reducing the tech debt and the number of bugs in comparison.
In this tutorial, We will learn the SOLID principles, which are the 5 most recommended design principles, that we should keep in mind while writing our classes.
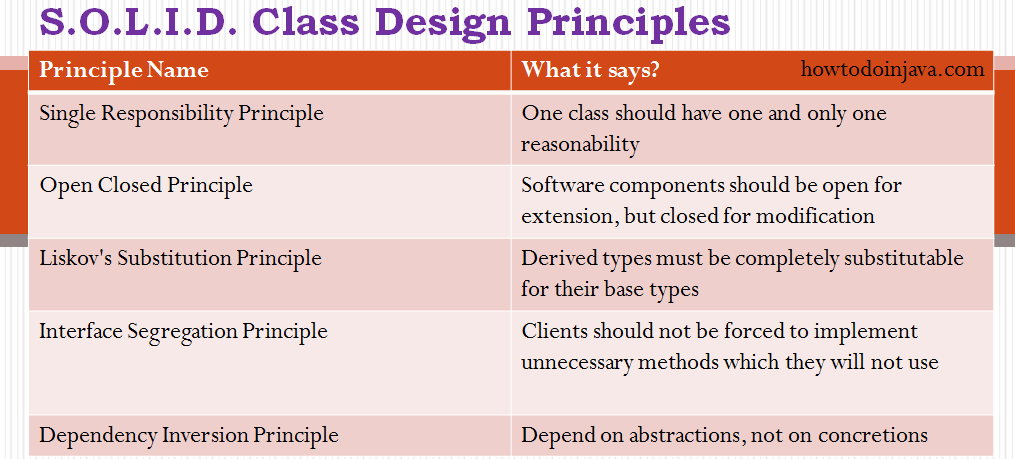
1. Introduction
SOLID is the acronym for a set of practices that, when implemented together, make the code more adaptive to change. Bob Martin and Micah Martin introduced these concepts in their book ‘Agile Principles, Patterns, and Practices’.
The acronym was meant to help us remember these principles easily. These principles also form a vocabulary we can use while discussing with other team members or as a part of technical documentation shared in the community. SOLID principles form the fundamental guidelines for building object-oriented applications that are robust, extensible, and maintainable.
2. Single Responsibility Principle [SRP]
We may come across one of the principles of object-oriented design, Separation of Concerns (SoC), that conveys a similar idea. The name of the Single Responsibility Principle says it all:
“One class should have one and only one responsibility”
In other words, we should write, change, and maintain a class only for one purpose. A class is like a container. We can add any amount of data, fields, and methods to it. However, if we try to achieve too much through a single class, soon that class will become bulky. If we follow SRP, the classes will become compact and neat, where each class is responsible for a single problem, task, or concern.
For example, if a given class is a model class then it should strictly represent only one actor/entity in the application. This kind of design decision will give us the flexibility to make changes in the class, in the future without worrying about the impacts of changes in other classes.
Similarly, If we are writing a service/manager class then the class should contain only that part of methods and nothing else. The service class should not contain even utility global functions related to the module.
Better to separate the global functions into another globally accessible class. This will help maintain the class for that particular purpose, and we can decide the visibility of the class to a specific module only.
Example
We can find plenty of classes in all popular Java libraries which follow the single responsibility principle. For example, in Log4j2, we have different classes with logging methods, different classes are logging levels and so on.
In our application-level code, we define model classes to represent real-time entities such as Person, Employee, Account etc. Most of these classes are examples of the SRP principle because when we need to change the state of a person, only then we will modify the Person class, and so on.
In the given example, we have two classes Person
and Account
. Both have single responsibility to store their specific information. If we want to change the state of Person then we do not need to modify the class Account and vice-versa.
public class Person {
private Long personId;
private String firstName;
private String lastName;
private String age;
private List<Account> accounts;
}
3. Open-Closed Principle
The open/closed principle is the second principle we should consider while designing our application. It states:
“Software components should be open for extension, but closed for modification”
It means that the application classes should be designed in such a way that whenever fellow developers want to change the flow of control in specific conditions in the application, all they need to do is extend the class and override some functions, and that’s it.
If other developers cannot write the desired behavior due to constraints put by the class, then we should reconsider refactoring the class. I do not mean here that anybody can change the whole logic of the class, but one should be able to override the options provided by the software in a nonharmful way permitted by the software.
Example
If we take a look into any good framework like struts or spring, we will see that we can not change their core logic and request processing. Still, we modify the desired application flow by extending some classes and plugin them in configuration files.
For example, spring framework has class DispatcherServlet
. This class acts as a front controller for Spring-based web applications. To use this class, we are not required to modify this class. All we need is to pass initialization parameters, and we can extend its functionality the way we want.
Please note that apart from passing initialization parameters during application startup, we can also override methods to modify the behavior of the target class by extending the classes. For example, struts Action classes are extended to override the request processing logic.
public class HelloWorldAction extends Action {
@Override
public ActionForward execute(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
//Process the request
}
}
4. Liskov’s Substitution Principle
LSP is a variation of the previously discussed open-closed principle. It says:
“Derived types must be completely substitutable for their base types”
LSP means that the classes, fellow developers created by extending our class, should be able to fit in the application without fail. This is important when we resort to polymorphic behavior through inheritance.
This requires the objects of the subclasses to behave the same way as the superclass objects. This is mostly seen in places where we do runtime type identification and then cast it to the appropriate reference type.
Example
An example of LSP can be custom property editors in Spring framework. Spring provides property editors to represent properties differently than the object itself e.g. parsing human-readable inputs from HTTP request parameters or displaying human-readable values of pure java objects in the view layer e.g. Currency
or URL
.
Spring can register one property editor for one data type and it is required to follow the constraint mandated by base class PropertyEditorSupport
. So if any class extends PropertyEditorSupport
class, then it can be substituted by everywhere the base class is required.
For example, every book has an ISBN number which is always in a fixed display format. You can have separate representations of ISBN in the database and UI. For this requirement, we may write property editor in such a way –
import java.beans.PropertyEditorSupport;
import org.springframework.util.StringUtils;
import com.howtodoinjava.app.model.Isbn;
public class IsbnEditor extends PropertyEditorSupport {
@Override
public void setAsText(String text) throws IllegalArgumentException {
if (StringUtils.hasText(text)) {
setValue(new Isbn(text.trim()));
} else {
setValue(null);
}
}
@Override
public String getAsText() {
Isbn isbn = (Isbn) getValue();
if (isbn != null) {
return isbn.getIsbn();
} else {
return "";
}
}
}
5. Interface Segregation Principle
This principle is my favorite one. ISP is applicable to interfaces as a single responsibility principle holds to classes. ISP says:
“Clients should not be forced to implement unnecessary methods which they will not use”
Take an example. Developer Alex created an interface Reportable
and added two methods generateExcel()
and generatedPdf()
. Now client ‘A’ wants to use this interface but he intends to use reports only in PDF format and not in excel. Will he be able to use the functionality easily?
NO. He will have to implement both methods, out of which one is an extra burden put on him by the designer of the software. Either he will implement another method or leave it blank. This is not a good design.
So what is the solution? The solution is to create two interfaces by breaking the existing one. They should be like PdfReportable
and ExcelReportable
. This will give the flexibility to users to use only the required functionality only.
Example
The best place to look for IPS examples is Java AWT event handlers for handling GUI events fired from keyboard and mouse. It has different listener classes for each kind of event. We only need to write handlers for events, we wish to handle. Nothing is mandatory.
Some of the listeners are –
- FocusListener
- KeyListener
- MouseMotionListener
- MouseWheelListener
- TextListener
- WindowFocusListener
Anytime, we wish to handle any event, find out a corresponding listener and implement it.
public class MouseMotionListenerImpl implements MouseMotionListener
{
@Override
public void mouseDragged(MouseEvent e) {
//handler code
}
@Override
public void mouseMoved(MouseEvent e) {
//handler code
}
}
6. Dependency Inversion Principle
Most of us are already familiar with the words used in the principle name. DI principle says:
“Depend on abstractions, not on concretions”
In other words. we should design our software so that various modules can be separated from each other using an abstract layer to bind them together.
Example
The classical use of this principle of bean configuration
in Spring framework.
In the spring framework, all modules are provided as separate components that can work together by injecting dependencies in other modules. This dependency is managed externally in XML files.
These separate components are so well closed in their boundaries that we can use them in other software modules apart from spring with the same ease. This has been achieved by dependency inversion and open-closed principles. All modules expose only abstraction, which is useful in extending the functionality or plug-in in another module.
7. Conclusion
These were 5 class design principles, also known as SOLID principles, which make the best practices to be followed to design our application classes and interfaces. When followed carefully, our systems design is easy to maintain and change overtime.
Happy Learning !!
Comments