In Java, we cannot override private, static and final methods declared in the parent class into the child classes. For private and final methods, the compiler will give errors. But in case of static methods, compiler allows to create methods with the same name and arguments.
Declaring similar static methods in parent and child classes is referred to as method hiding. For non-static methods, it is known as method overriding.
1. Understanding Method Hiding with an Example
In the following code, we have created two classes Parent and Child. Both classes define a static method display(). This code complies successfully without any warning or error.
class Parent {
static void display() {
System.out.println("Super");
}
}
class Child extends Parent {
static void display() {
System.out.println("Sub");
}
}
At first sight, it looks like that Child has overridden the display() method from the Parent class. If this is true, then the following program should print “Sub” according to the rules of method overriding.
Parent instance = new Child();
instance.display(); //Prints Super
2. Can We Override Static Methods by Declaring Non-static in Child Class?
No, we cannot remove the static keyword from the method declaration in the child class. The compiler will give an error in such an attempt.
In the previous example, if we remove the static keyword from display() method in Child, the compiler complains that we can not override a static method from Parent.
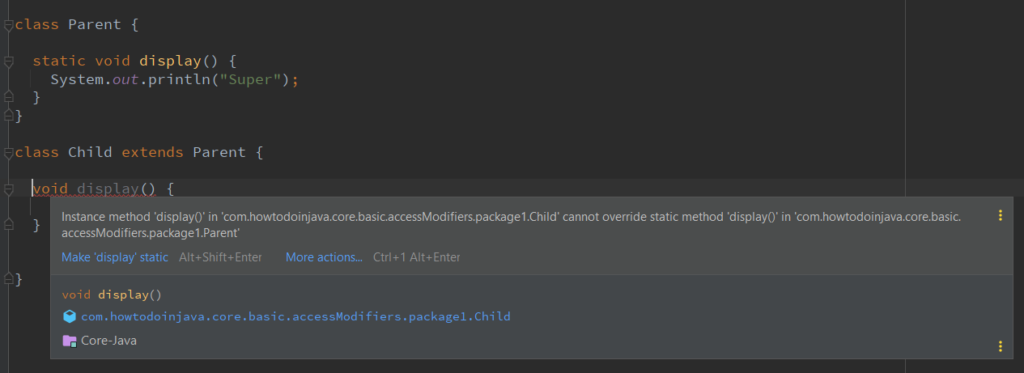
3. Conclusion
In Java, method overriding is valid only when we are talking in terms of instance methods. As soon as, we start talking in terms of static methods, the term should be used is method hiding, because static methods belong to the class object.
Fortunately, the above terms are in most Java literature (even in Java Language Specification).
Happy Learning !!
Comments