Java has been cited to be a very verbose language for even a simple hello world program and small proof-of-concept works. Since Java 21, we can use unnamed classes and instance main methods that allow us to bootstrap a class with minimal syntax.
This change will benefit mostly the beginners who have just started to learn Java and want to try out the language syntax for quick learning.
1. Changes at a Glance
The following are the highlights of this change under JEP-445. Note that
- No class declaration is required
- Java keywords
public
andstatic
are no longer required - Main method argument
args
is optional
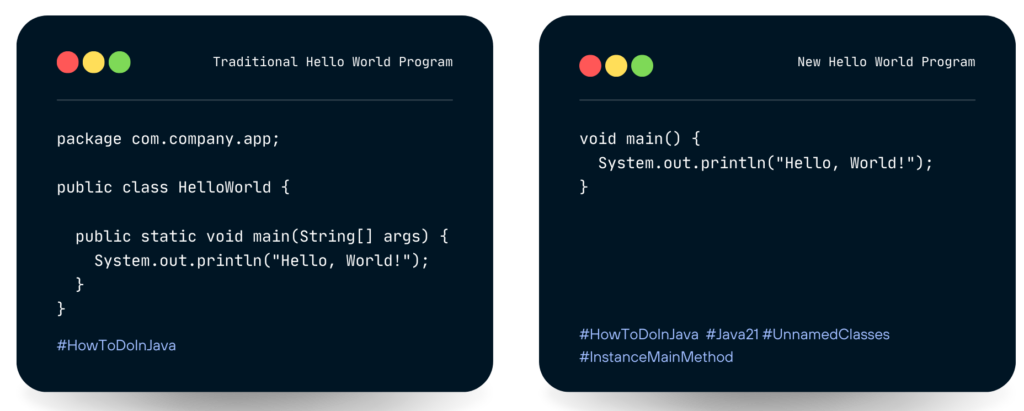
Prior to Java 21, we had to write the following class to execute a simple “Hello, World!” message:
package com.company.app;
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Since Java 21, the following code is a fully functional class that will print the output “Hello, World!” to the console. We can store this class in any Java file such as HelloWorld.Java and then we can run it.
void main() {
System.out.println("Hello, World!");
}
This is a preview language feature in Java 21, disabled by default. Use --enable-preview
flag to use this feature.
$ java.exe --enable-preview --source 21 HelloWorld.Java
// Prints
Hello, World!
2. Instance Main Methods: Flexible Ways to Write main() Methods
Since Java 21, Java compiler/runtime will not force us to write a strict matching syntax for the main() method though if it is still allowed to write it in an unnamed class.
This means we can write more than main() method in a class. The Java compiler will search the main() method in the following sequence. Whatever the JVM finds the first main() method in the sequence, it will use it to launch the program:
- A
static void main(String[] args)
method - A
static void main()
method without any arguments - A
void main(String[] args)
instance method withoutstatic
keyword - A
void main()
instance method withoutstatic
keyword and arguments
Note that, in all above cases, the method must be non-private i.e. it must have access modifier either public
, protected
or package
.
For example, in the following program, we have created two main methods.
void main(String[] args) {
System.out.println("Main method with args");
}
void main() {
System.out.println("Main method without args");
}
In the sequence, the void main(String[] args) comes before the void main(), so the program output will be:
Main method with args
Note that the above instance main() methods can be written with normal classes also. The following Java program is a valid program and it will execute the main()
method.
public class Main {
void main() {
//...
}
}
3. Rules to Create and Use an Unnamed Class
There are certain rules to abide by to create the unnamed classes correctly. Let us check them out.
3.1. The ‘package’ Statement is Not Allowed
An unnamed class is always a member of the unnamed package. So we cannot declare a package statement in it.
package com.howtodoinjava.java21;
void main() {
System.out.println("Hello, World!");
}
The program output:
Main.java:1: error: unnamed class should not have package declaration
package com.howtodoinjava.java21;
^
3.2. No Constructor is Allowed
Since we cannot refer to the unnamed class by its name, we cannot create a constructor as well.
It also means that we cannot create an instance of an unnamed class. That is why these classes are suited only for simple POC and learning purposes.
3.3. A main() Method must Exist
Since we cannot create a direct instance of an unnamed class, to make it usable, it must have a main() method to execute its code. Java compiler will throw an error if there is no main method.
void display() {
System.out.println("Hello, World!");
}
The Java compiler will fail with the following error:
Main.java:4: error: unnamed class does not have main method in the form of void main() or void main(String[] args)
void display() {
^
...
error: compilation failed
extend
or implement
3.4. Unnamed class cannot An unnamed class is always final
and it cannot extend another class (except Object) or implement an interface.
The absence of a class name simply does not allow to use of the syntax to extend or implement anything.
3.5. Access to Static Members
Since unnamed classes cannot be referenced with names, we cannot reference their static methods and variables with the class name. We can either access them directly or use the this
keyword.
static void main() {
if(staticVariable) {
System.out.println("static variable");
}
staticMethod();
}
static boolean staticVariable = true;
static void staticMethod(){
System.out.println("static method");
}
The program output:
static variable
static method
4. Conclusion
Java 21 unnamed classes feature is a great addition to the language which will benefit the programmer who inspires to learn Java and come from other languages with similar quick-to-start syntaxes, such as Python. Similarly, instance main() methods will help in reducing the ceremonial bulk from simple Java programs intended to learn the language basics and simple proof works.
Happy Learning !!
Comments