In this post, we are going to discuss thread priorities in detail and the different types of thread priorities in Java, and how a thread scheduler executes various threads based on their priorities. We will also see how we can set thread priority of a thread and how we can get the priority of an existing thread.
1. Introduction to Thread Priority
Every Thread in java has a priority. It may be the default priority assigned by the JVM or a customized priority explicitly provided by the programmer. The valid range of thread Priority is between 1 to 10, where 1 is the minimum and 10 is the maximum priority. The default priority is 5.
In a multi-threaded environment, the thread scheduler uses the priorities while allocating processors to the threads for their execution.
- The Thread having the highest priority will be executed first followed by other low priority threads waiting for their execution. This means the scheduler gives preference to high-priority threads.
- If multiple threads are having same priority, then the execution order of the threads will be decided by the thread scheduler based on some internal algorithm, either round robin, preemptive scheduling, or FCFS (First Come First Serve) algorithm.
- The thread scheduler is part of the JVM and varies from JVM to JVM and hence we can’t expect the exact algorithm used by the scheduler.
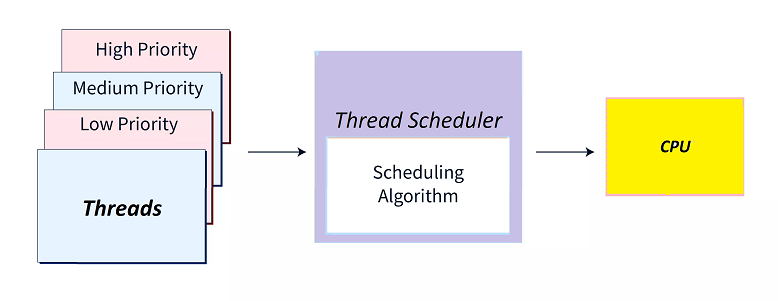
See Also: Thread Life Cycle
2. Types of Thread Priority
The Thread class contains 3 constants to define thread priorities:
- MIN_PRIORITY: This is the minimum priority with the value of 1.
- NORM_PRIORITY: This is the default normal priority with the value of 5.
- MAX_PRIORITY: This is the maximum priority with the value of 10.
public static int MIN_PRIORITY
public static int NORM_PRIORITY
public static int MAX_PRIORITY
3. Working with Thread Priority
Let’s now look at how we can use thread priority in our code and how we can change the priority of a thread as per the requirement.
3.1. Getting and Setting Thread Priority
We can use the following methods to get and set the priority of Thread:
int getPriority()
: returns the priority of the given thread.void setPriority(int newPriority)
: sets a new priority for a thread. The valid range for passing new priority value as argument to this method is between 1 to 10. If we pass a value outside of this range, then this method throws ‘IllegalArgumentException’.
3.2. Thread Priority Example
Let’s now look at an example explaining the use of thread priorities. For this example, we are creating a new thread by extending Thread
class and then perform get & set operations for thread priorities.
// Creating 3 Threads
Thread thread1 = new Thread();
Thread thread2 = new Thread();
Thread thread3 = new Thread();
// Getting Priorities of above threads
System.out.println("Thread1 priority : " + thread1.getPriority()); // Print ‘5’ as default priority
System.out.println("Thread2 priority : " + thread2.getPriority()); // Print ‘5’ as default priority
System.out.println("Thread3 priority : " + thread3.getPriority()); // Print ‘5’ as default priority
// Setting priority for above threads
thread1.setPriority(4);
// Setting Priority of 1 using Thread constant for minimum priority
thread2.setPriority(Thread.MIN_PRIORITY);
// thread3.setPriority(15); // This will throw IllegalArgumentException
// Again getting Priorities for above Threads
System.out.println("Thread1 new priority : " + thread1.getPriority()); // Print ‘4’ as new priority
System.out.println("Thread2 new priority : " + thread2.getPriority()); // Print ‘1’ as new priority
3.3. Thread Priority of Main Thread
The main thread is having a default priority of ‘5’. We can change the priority of the main thread by using setPriority()
class.
class MainThreadDemo extends Thread
{
public static void main(String[] args)
{
// Getting priority for main thread
System.out.println(Thread.currentThread().getPriority());
// Print ‘5’ as default priority
// Setting priority for main thread
Thread.currentThread().setPriority(Thread.MAX_PRIORITY);
// Getting priority for main thread again
System.out.println(Thread.currentThread().getPriority()); // Print ‘10’ as new priority
}
}
4. Thread Priority w.r.t. Parent-Child Relationship
When we don’t provide any priority for a thread, then main thread will be having a default priority of 5. And for all the remaining threads, the default priority inherits from the parent thread to the child thread.
That means that whatever priority the parent thread has, the same priority is inherited for the child thread as well.
class ThreadDemo extends Thread
{
public void run()
{
System.out.println(“Inside run method”);
}
public static void main(String[] args)
{
// Setting priority for main thread
Thread.currentThread().setPriority(7);
// Printing main thread priority
System.out.println("Main thread priority: " + Thread.currentThread().getPriority());
// Creating child thread
ThreadDemo childThread = new ThreadDemo();
// Printing child thread priority
System.out.println("Child thread priority: " + childThread.getPriority()); //7
}
}
Notice the program output. We can see that both the parent thread (main) and the child thread (childThread) have the same priorities. Even though we haven’t set the priority of the child thread, it is picking the same priority as its parent thread (main thread in this case).
5. Executing Threads with the Same Priority
We know that the thread scheduler generally executes high priority threads first before executing low priority threads. We can have a scenario where 2 threads are having same priority, so in this case we can’t guess which thread executes first. It totally depends on the internal algorithm used by the thread scheduler for scheduling such threads.
Let’s cover an example for this scenario as well.
public class ThreadPriorityDemo {
public static void main(String[] args) {
// Creating child tasks
ChildTask childTask1 = new ChildTask();
ChildTask childTask2 = new ChildTask();
// Start Child Threads
new Thread(childTask1).start();
new Thread(childTask2).start();
System.out.println(Thread.currentThread().getName() + " executed by main thread");
}
}
class ChildTask implements Runnable {
public void run()
{
System.out.println(Thread.currentThread().getName() + " executed by child thread");
}
}
In this example, both the parent thread (main thread) and the child thread (thread1) are having same priority of ‘5’. Notice the program output below. We can see 2 possible outputs for this scenario as the scheduler varies from system to system and can execute any thread (main or child thread) first as both are having the same priority.
main executed by main thread
Thread-1 executed by child thread
Thread-0 executed by child thread
6. Conclusion
We have covered thread priorities and their types in java and how we can get and set a thread priority for various threads with practical examples.
We have also examined scenarios where multiple threads are having the same priority and how thread priority behaves w.r.t the parent-child thread relationship.
Happy Learning !!
Comments