JUnit 5 @Tag is used to tag or label the tests into a test suite. It helps organize and filter the tests based on different criteria or attributes, such as their purpose, the features they test, or their characteristics. This particularly helps in selectively running a subset of tests based on their tags, making test suite management more flexible.
We can execute a set of tests by including only those tagged tests in the test plan OR by excluding other tests from the test plan.
1. Using @Tag Annotation
We can apply the @Tag
annotation on a test class, test method, or both. We can use any string as a tag value, but it’s common to use descriptive tags like “smoke,” “regression,” “integration,” “security,” and so on.
@Tag("development")
public class ClassATest
{
@Test
@Tag("userManagement")
void testCaseA(TestInfo testInfo) {
}
}
We can apply multiple tags on a single test case as well so that you can include the test in multiple test suites.
public class ClassATest
{
@Test
@Tag("development")
@Tag("production")
void testCaseA(TestInfo testInfo) {
}
}
2. Creating Test Suites with @IncludeTags and @ExcludeTags
We can use @IncludeTags or @ExcludeTags annotations in the suites to filter tests or include tests.
Take an example. In the following example, when the first suite runs then it will run all the tests tagged with “production” inside the package ‘com.howtodoinjava.junit5.examples‘. In the second example, we exclude all those tests and execute the remaining tests.
//@IncludeTags example
@Suite
@SelectPackages("com.howtodoinjava.junit5.examples")
@IncludeTags("production")
public class MultipleTagsExample
{
}
//@ExcludeTags example
@Suite
@SelectPackages("com.howtodoinjava.junit5.examples")
@ExcludeTags("production")
public class MultipleTagsExample
{
}
To add more than one tag, pass a string array of tags in the desired annotation.
@Suite
@SelectPackages("com.howtodoinjava.junit5.examples")
@IncludeTags({"production","development"})
public class MultipleTagsExample
{
}
We CAN NOT include both
@IncludeTags
and@ExcludeTags
annotations in the same test suite.
3. Running Tagged Tests
3.1. In Maven
To run tests with specific tags, we can use the -groups
and -excludeGroups
options when executing tests with a build tool (e.g., Maven or Gradle) or an IDE.
To run the tests with specific tags using Maven, use the following command:
mvn test -Dgroups="localhost,development"
To exclude the tagged tests, we run the following command:
mvn test -DexcludeGroups="production"
You can also configure the tags via the configuration parameters of the maven-surefire-plugin
.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.1.2</version>
<configuration>
<!-- include tags -->
<groups>localhost,development</groups>
<!-- exclude tags -->
<excludedGroups>production</excludedGroups>
</configuration>
</plugin>
Then run the test using the regular ‘mvn test‘ command.
mvn clean test
3.2. In Gradle
To achieve the equivalent of running tests with specific groups (tags) in Gradle, you can use the --tests
and --include-tags
options.
In this example, it includes tests with the tags “localhost” and “development.” The --tests *
part is a wildcard to indicate that you want to run all test classes.
gradle test --tests * --include-tags=localhost --include-tags=development
Similarly, to execute the tagged tests we can run the following command. In this example, it excludes tests with the “production” tag.
gradle test --tests * --exclude-tags=production
To configure the tagged tests to include or exclude in build.gradle, we can provide the following configuration:
test {
useJUnitPlatform()
includeTags 'localhost', 'development'
excludeTags 'production'
}
3.3. In IDE (IntelliJ)
Several IDEs support running the tagged test cases through custom run configuration. For example, in IntelliJ, we can create a custom run configuration and select Tags in in type of resources to search.
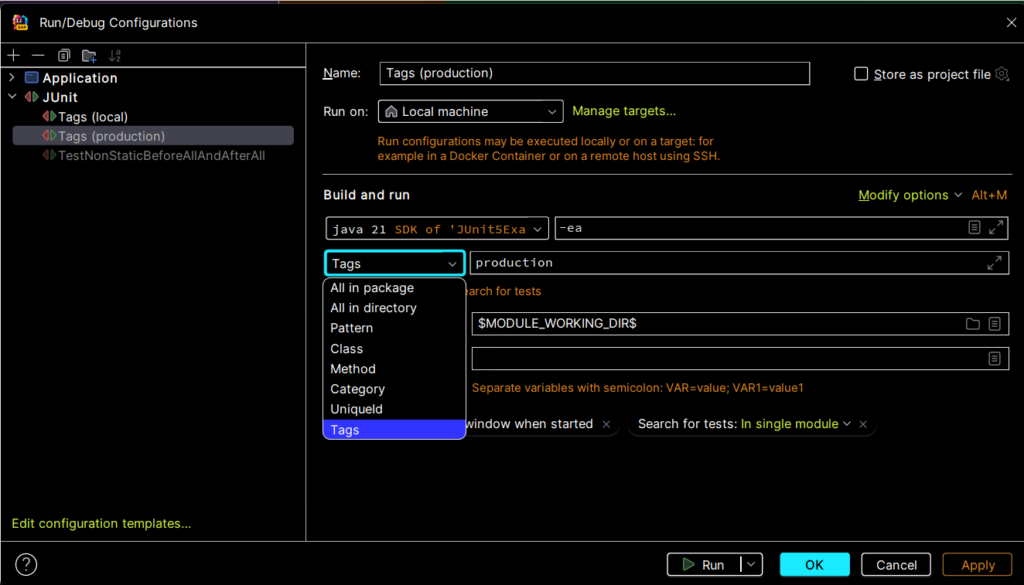
4. JUnit 5 @Tag Example
Let’s say we have 3 tests and we want to run all 3 in the development environment but want to run only one in production. So we will tag the tests as below:
public class ClassATest
{
@Test
@Tag("development")
@Tag("production")
void testCaseA(TestInfo testInfo) { //run in all environments
}
}
public class ClassBTest
{
@Test
@Tag("development")
void testCaseB(TestInfo testInfo) {
}
}
public class ClassCTest
{
@Test
@Tag("development")
void testCaseC(TestInfo testInfo) {
}
}
Let’s create two test suites – one for each environment.
Tests to run in the production environment
@Suite
@SelectPackages("com.howtodoinjava.junit5.examples")
@IncludeTags("production")
public class ProductionTests
{
}
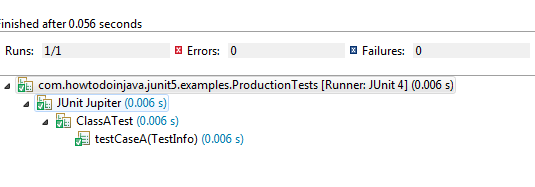
Tests to run in the development environment
@Suite
@SelectPackages("com.howtodoinjava.junit5.examples")
@IncludeTags("development")
public class DevelopmentTests
{
}
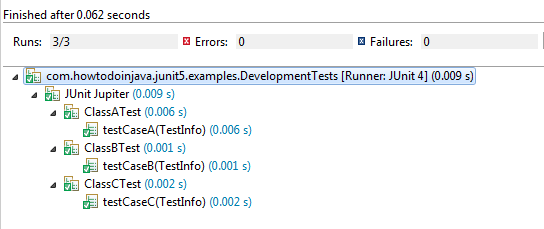
Happy Learning !!
Comments