In Python, list comprehension is a concise way to create a new list based on the values of an existing list. List comprehension is often used for its brevity and readability compared to traditional for-loop.
This Python tutorial discusses what is list comprehension, and its syntax with easy-to-understand examples, using if-else style conditions in comprehensions and writing nested list comprehensions involving two lists.
# A new list of even numbers from existing list containing numbers 0-9
even_numbers = [x for x in range(10) if x % 2 == 0]
print(even_numbers) # [0, 2, 4, 6, 8]
# If-else with list comprehension
labels = ["Even" if x % 2 == 0 else "Odd" for x in range(10)]
print(labels)
# Two Lists Comprehension
list1 = [1, 2, 3]
list2 = [3, 2, 1]
combined = [(x, y) for x in list1 for y in list2 if x != y]
print(combined)
1. Syntax
The basic syntax of list comprehension is:
[expression for item in iterable if condition]
This syntax includes:
expression
: The output expression producing elements of the new listitem
: The variable representing members of the input sequenceiterable
: A sequence, collection, or an iterator objectcondition
: (Optional) A condition to filter items from the input sequence
We can map the syntax parts to the previous example as follows:
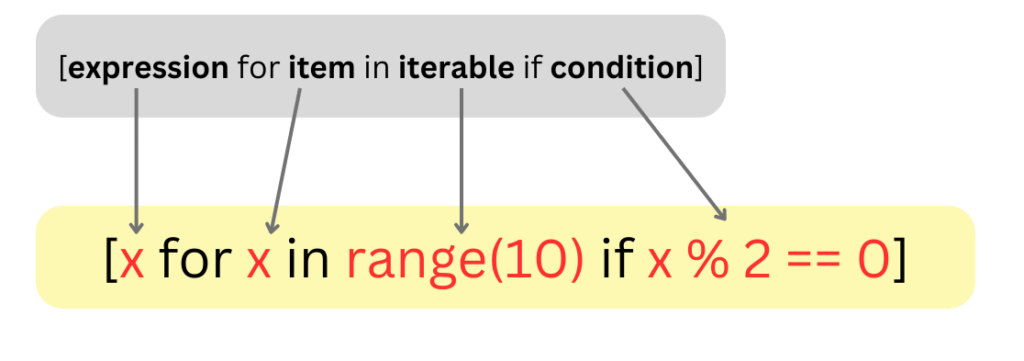
For example, to get even numbers from a list, we can write a simple for-loop and its equivalent list comprehension as follows:
even_numbers = []
for x in range(10):
if x % 2 == 0:
even_numbers.append(x)
print(even_numbers) # [0, 2, 4, 6, 8]
even_numbers = [x for x in range(10) if x % 2 == 0]
print(even_numbers) # [0, 2, 4, 6, 8]
2. List Comprehension Examples
It is always easy to understand what we can do with concise syntaxes with more and more examples. So let’s check out some examples.
2.1. Squaring Numbers
In the following list comprehension example, we are creating a list of squares for numbers from 0 to 4.
squares = [x**2 for x in range(5)]
print(squares) # [0, 1, 4, 9, 16]
2.3. Lowercase Conversion
In the following list comprehension example, we are converting all the words in a list to lowercase.
words = ["List", "COMPREHENSIONS", "Are", "COOL"]
lower_words = [word.lower() for word in words]
print(lower_words) # ['list', 'comprehensions', 'are', 'cool']
2.4. Extracting Numbers from a String
In the following list comprehension example, we are extracting all the digits from a string and creating a list of integers.
string = "Hello 12345 World"
numbers = [int(s) for s in string if s.isdigit()]
print(numbers) # [1, 2, 3, 4, 5]
2.5. Flattening a Matrix
The example is to convert a matrix (list of lists) into a flat list.
matrix = [[1, 2], [3, 4], [5, 6]]
flattened = [num for row in matrix for num in row]
print(flattened) # [1, 2, 3, 4, 5, 6]
2.6. Generating Random Numbers
In the following example, we are creating a list of random numbers greater than 0.5. Note that the output will differ in each run as the number of random numbers generated (greater than 0.5) in sequence may differ each time.
import random
numbers = [random.random() for _ in range(10)]
randoms = [x for x in numbers if x >= 0.5]
print(randoms) # [0.6296657207360175, 0.9508260559890607, 0.6601432602022057]
3. List Comprehension using If-else
We use an if-else
statement within a list comprehension expression. This allows us to choose between two possible outcomes for each item in the iterable. It’s a useful feature for cases where we need to apply different transformations or labels to the elements of a list depending on certain conditions.
The syntax for including an if-else
statement in a list comprehension is as follows. In this syntax, expression_if_true is evaluated and included in the new list if the condition is true for the item, otherwise, expression_if_false is evaluated and included.
[expression_if_true if condition else expression_if_false for item in iterable]
For example, in the following code, we are creating a list that labels numbers from another list as ‘Even’ or ‘Odd’. The second list produces numbers 0 to 9.
labels = ["Even" if x % 2 == 0 else "Odd" for x in range(10)]
print(labels)
The program output:
['Even', 'Odd', 'Even', 'Odd', 'Even', 'Odd', 'Even', 'Odd', 'Even', 'Odd']
4. Two Lists Comprehension – Nested For-Loops
Double list comprehension, or nested list comprehension, involves one list comprehension within another. This technique is useful for processing and creating multi-dimensional lists or matrices.
Be cautious because the double list comprehension works like a nested for loop so it quickly generates a lot of results.
In the following example, we are combining the elements from two lists if they are not equal.
list1 = [1, 2, 3]
list2 = [3, 2, 1]
combined = [(x, y) for x in list1 for y in list2 if x != y]
print(combined)
The program output:
[(1, 3), (1, 2), (2, 3), (2, 1), (3, 2), (3, 1)]
Due to a tendency to quickly become unreadable, using list comprehensions within list comprehensions is not advisable.
4. Conclusion
List comprehension in Python provides an efficient way to create new lists without using temporary variables. It enhances readability and often performance. However, it’s important to balance its use with code readability, especially in cases of complex expressions.
Happy Learning !!
Comments