In TypeScript, the array.slice() method extracts a section of an array (slice of an array) and returns it as a new array. It allows us to create a new array containing a shallow copy of the selected elements from the source array without modifying the source array.
const array:string[] = ["a", "b", "c", "d", "e", "f", "g"];
let subarray:string[];
//Original array is returned if no argument
subarray = array.slice(); //"a", "b", "c", "d", "e", "f", "g"]
//slicing begins from this index (inclusive)
subarray = array.slice(3); //["d", "e", "f", "g"]
//slicing in range from begin (inclusive) to end (exclusive)
subarray = array.slice(3, 6); //["d", "e", "f"]
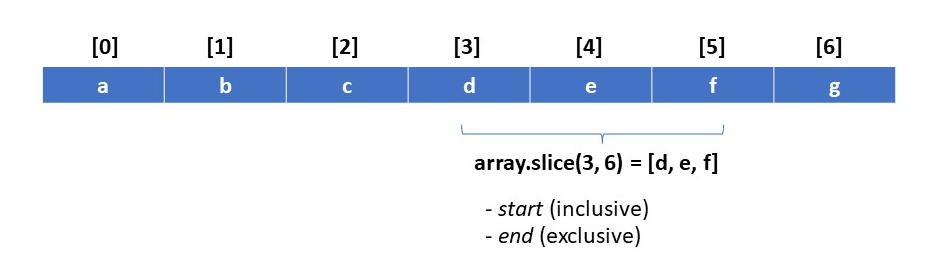
1. Array.slice() Syntax
The slice() method takes two optional parameters: start and end. By default the start is 0 and end is array.length.
Array.slice(start?: number | undefined, end?: number | undefined):
- start (inclusive): The beginning index of the array.
- If
start
is undefined, then the slice begins at index 0. - A negative value specifies an array element measured from the end of the array.
- If
- end (exclusive): The end index of the array.
- If
end
is undefined, then the slice extends to the end of the array. - A negative value specifies an array element measured from the end of the array.
- If
The slice() method returns a new array that contains the elements of the original array from the element specified by start
, up to, but not including, the element specified by end
.
The slice() does not modify the original array. If we want to remove a slice from the original array, use Array.splice().
2. Array.slice() contains Shallow Copies of Array Elements
It is important to understand that, although slice() returns a new array, still the copied elements are a shallow copy of the elements in the source array. The rules for the shallow copy apply to the array elements:
- For string, number and boolean,
slice()
will copy the values to the new array. Changes in the copied array elements don’t affect the source array elements. - For object types, slice() will copy the reference of the objects to the new array. So, any change in the objects will be visible in both arrays.
In the following example, we are modifying the age of employee2. We can see that the changes are visible in both arrays.
let employee1 = { name: 'Alex', age: 30 };
let employee2 = { name: 'Bob', age: 31 };
let employee3 = { name: 'Charles', age: 32 };
let sourceArray = Array.of(employee1, employee2, employee3);
let sliceArray = sourceArray.slice(1,2);
//Change an object
sliceArray[0].age = 40;
//Change is visible in both arrays
console.log(sourceArray[1]); //[{ "name": "Bob","age": 40 }]
console.log(sliceArray); //[{ "name": "Bob","age": 40 }]
3. Array.slice() preserves Empty Slots
If the source array contains the empty slots then the sliced array also contains the empty slots if they fall in the range of the slicing.
const array = ["a", "b", , , "e", "f", "g"];
const subarray = array.slice(1, 5);
console.log(subarray); //["b", , , "e"]
4. Array.slice() with Negative Indices
The negative values of start and end positions are counted from the end of the array. It is the responsibility of the programmer to provide the correct index range to slice the array correctly.
A good way to ensure the valid range for slicing is that start should always be less than the end.
const array:string[] = ["a", "b", "c", "d", "e", "f", "g"];
subarray = array.slice(-1, -3); //[] - 'start' is greater than 'end'
subarray = array.slice(-3, -1); //["e", "f"] - 'start' is less than 'end'
Let us understand the above example with an image. We can see how the negative indices start from the end. All other rules still apply.
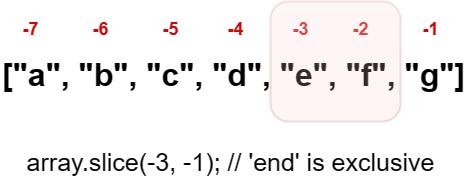
5. Conclusion
Typescript array slicing is an excellent function that can be used in data handling and analysis. The important thing to remember is that the array items are shallow copies of the items in the original array, so avoid changing the elements in the sliced array until the elements are primitive types.
Happy Learning !!
Comments