Learn to find the union between two arrays in Java using HashSet and Stream APIs. In set theory, the union (denoted by U) of a collection of sets is the set of all elements in the collection.
For example, the union of two arrays A and B is a collection of all the elements which are either in A, or in B, or in both A and B.
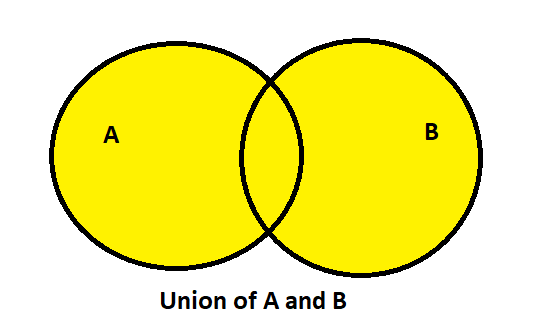
1. Union of Arrays using HashSet
To get the union of two arrays, follow these steps:
- Push the first array in a HashSet instance.
- Use addAll() method to add the elements of the second array into the set.
- Similarly, add all the elements of more arrays in the set, if any.
Java program to get the union between two integer arrays and print the output.
Integer[] arr1 = {0, 2};
Integer[] arr2 = {1, 3};
HashSet<Integer> set = new HashSet<>();
set.addAll(Arrays.asList(arr1));
set.addAll(Arrays.asList(arr2));
Assertions.assertEquals(Set.of(0, 1, 2, 3), set);
//convert to array, if needed
Integer[] union = set.toArray(new Integer[0]);
Assertions.assertArrayEquals(new Integer[]{0, 1, 2, 3}, union);
2. Using Java 8 Stream
We can use the Stream APIs to iterate over the elements of both streams and use flatMap() to normalize the items of both arrays in a single stream, and then collect to a new array.
Using Streams allows us to perform additional actions on the union items in the same stream pipeline, and collect the results in a variety of target types.
Integer[] arr1 = {0, 2}; Integer[] arr2 = {1, 3}; Integer[] union = Stream.of(arr1, arr2) .flatMap(Stream::of) .toArray(Integer[]::new); Assertions.assertArrayEquals(new Integer[]{0, 1, 2, 3}, union);
Happy Learning !!
Read More : How to get intersection of two arrays
Comments