Learn to create and handle custom exceptions using resteasy ExceptionMapper interface implementations. ExceptionMapper
is a contract for a provider that maps Java exceptions to Response
object.
An implementation of
ExceptionMapper
interface must be annotated with@Provider
to work correctly.
1. Resteasy ExceptionMapper – Custom exception handler
A sample implementation provider class of ExceptionMapper
looks like this:
package com.howtodoinjava.exception; import javax.ws.rs.core.Response; import javax.ws.rs.core.Response.Status; import javax.ws.rs.ext.ExceptionMapper; import javax.ws.rs.ext.Provider; @Provider public class MyApplicationExceptionHandler implements ExceptionMapper<MyApplicationException> { @Override public Response toResponse(MyApplicationException exception) { return Response.status(Status.BAD_REQUEST).entity(exception.getMessage()).build(); } }
Where the custom exception class MyApplicationException.java
is written as:
package com.howtodoinjava.exception; import java.io.Serializable; public class MyApplicationException extends Exception implements Serializable { private static final long serialVersionUID = 1L; public MyApplicationException() { super(); } public MyApplicationException(String msg) { super(msg); } public MyApplicationException(String msg, Exception e) { super(msg, e); } }
2. Resteasy REST API
To test the ExceptionMapper
implementation, I have written following resteasy REST API.
package com.howtodoinjava.rest; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.core.Response; import org.jboss.resteasy.spi.validation.ValidateRequest; import com.howtodoinjava.exception.MyApplicationException; @Path("/rest") public class UserService { @Path("/users/{id}") @GET @ValidateRequest public Response getUserBId ( @PathParam("id") String id ) throws MyApplicationException { //validate mandatory field if(id == null) { throw new MyApplicationException("id is not present in request !!"); } //Validate proper format try { Integer.parseInt(id); } catch(NumberFormatException e) { throw new MyApplicationException("id is not a number !!"); } //Process the request return Response.ok().entity("User with ID " + id + " found !!").build(); } }
3. RESTEasy ExceptionMapper demo
Above API accepts the user 'id'
parameter in Integer
format. If we pass the id in some other format which can not be parsed to Integer
, it will throw MyApplicationException
. Our exception mapper should be able to handle this.
3.1. Valid request
Access http://localhost:8080/RESTEasyExceptionMapperDemo/rest/users/1
in browser.
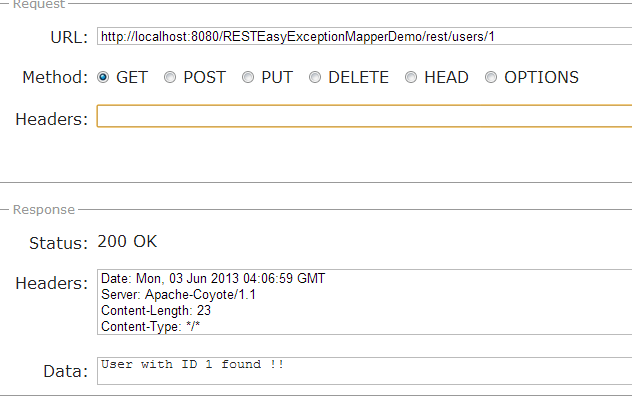
3.2. Invalid request – throws exception
Access http://localhost:8080/RESTEasyExceptionMapperDemo/rest/users/abc
in browser.
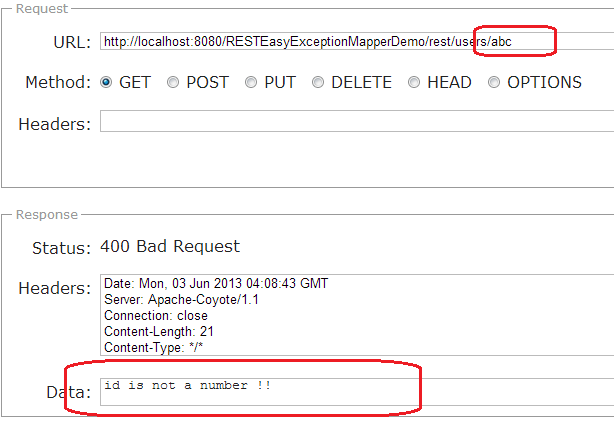
To download the sourcecode of this example of Resteasy client exception handling with ExceptionMapper inreface, follow below given link.
Happy Learning !!
Comments