In Spring Boot MVC applications, custom error pages help deliver a polished user experience when an error occurs during request processing. It helps to show informative and user-friendly error messages for different types of errors and HTTP status codes.
In this tutorial, we will learn to create custom error views for specific HTTP error codes in a Spring Boot application.
1. The Default Behavior
Spring Boot, by default, comes with error handling enabled and will show a default error page and stacktrace (also called a whitelabel page). This default error-handling behavior is provided by Spring Boot’s error-handling mechanism.
The default error page typically includes the following information:
- The HTTP status code of the error (e.g., 404 for Not Found, 500 for Internal Server Error).
- A brief description of the error.
- Optionally, a stack trace detailing the exception that occurred.
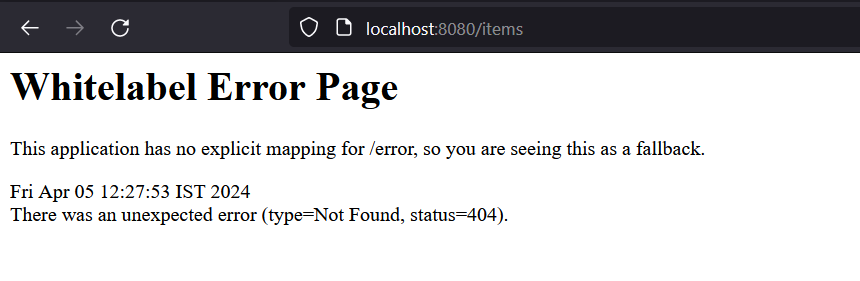
The default behavior can be disabled in full by setting the ‘server.error.whitelabel.enabled’ property to ‘false’.
server.error.whitelabel.enabled=false
We can also achieve this by excluding the ErrorMvcAutoConfiguration class that autoconfigures the default behavior.
@Configuration
@EnableAutoConfiguration(exclude = {ErrorMvcAutoConfiguration.class})
public class WebMvcConfig {
}
When disabled, the exception handling will be handled by the servlet container instead of the general exception handling mechanism provided by Spring and Spring Boot.
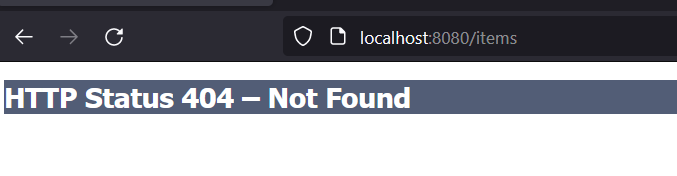
2. Including or Excluding the Exception Information
In a real-world application, we may want to include the exception information for debugging purposes, but we may want to exclude it in a production environment to avoid exposing sensitive information to users.
These are some properties that can be used to configure the whitelabel error page, mainly for what is going to be included in the model.
- server.error.whitelabel.enabled: Sets whether the whitelabel error page is enabled; the default is true.
- server.error.path: Sets the path of the error page; the default is /error.
- server.error.include-exception: Sets whether the name of the exception (e.g., java.lang.NullPointerException) should be included in the model; the default is false.
- server.error.include-stacktrace: Sets whether the stack trace should be included in the model; the default is NEVER. Possible values are NEVER, ALWAYS, ON_WHITELABEL.
- server.error.include-message: Sets whether the message should be included in the model; the default is NEVER. Possible values are NEVER, ALWAYS, ON_WHITELABEL.
- server.error.include-binding-errors: Sets whether the message should be included in the model; the default is NEVER. Possible values are NEVER, ALWAYS, ON_WHITELABEL.
For example, if you now set ‘server.error.include-exception’ to true and ‘server.error.include-stacktrace’ and ‘server.error.include-message’ to always, the customized error page will show the classname of the exception, the message, and the stacktrace.
server.error.include-exception=true
server.error.include-message=ALWAYS
server.error.include-stacktrace=ALWAYS
For example, let’s add a method to a RestController class that forces an exception.
@GetMapping("/records/500")
public void error() {
var cause = new NullPointerException("Dummy Exception");
throw new ServerErrorException(cause.getMessage(), cause);
}
Now check the same error page again after accessing the URL: ‘/records/500‘
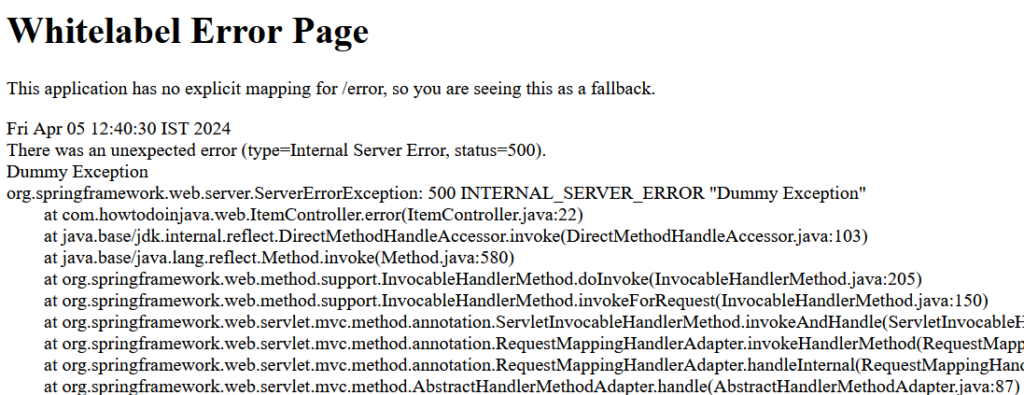
3. Serving Custom Error Pages
To serve the custom error views, we configure the application to handle specific error conditions and map them to custom error views. For example, we can add an error.html file as a customized generic error page, or specific error pages for specific HTTP error codes, i.e., 404.html and 500.html in the ‘src/main/resources/templates’ directory.
3.1. Default Error Page (error.html)
To configure a default error page for the /error
path, create a default error page HTML file (e.g., error.html
) in the src/main/resources/templates
directory of the Spring Boot project. This file will serve as the default error page template.
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>HowToDoInJava Error Page</title>
</head>
<body>
<h1>Oops, something went wrong!</h1>
<div>
<div>
<span><strong>Status</strong></span>
<span th:text="${status}"></span>
</div>
<div>
<span><strong>Error</strong></span>
<span th:text="${error}"></span>
</div>
</div>
</body>
</html>
The location may be different based on the template provider. The default is Thymleaf which picks the resources from ‘/template‘ directory.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
Note that, by default, Spring Boot maps the
/error
path to handle error responses. So do not map this path in other APIs or methods.
Now we can test that, by default, this page is shown for all errors.
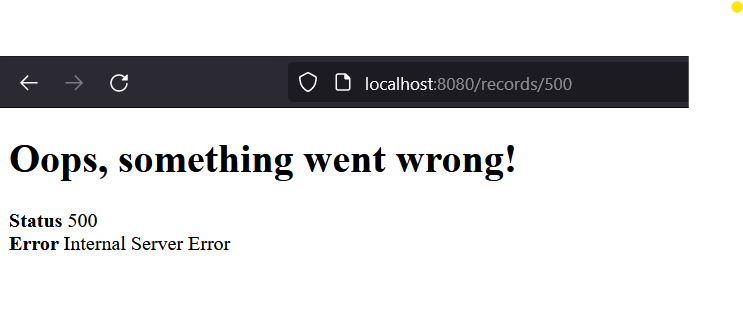
3.2. HTTP Status-specific Error Page (4xx.html and 5xx.html)
We can be more specific for specific error codes to be more user-friendly. Such as we can show a custom page for 404 errors (page not found) and another page for 500 errors (internal server error). This makes the user realize his mistake more effectively.
We can add these pages (404.html, 500.html, and other such pages specific to HTTP status codes) in the ‘/templates/error‘ directory.
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>HowToDoInJava 404 Page</title>
</head>
<body>
<h1>Oops, Page Not Found!</h1>
<div>
<div>
<span><strong>Status</strong></span>
<span th:text="${status}"></span>
</div>
<div>
<span><strong>Error</strong></span>
<span th:text="${error}"></span>
</div>
</div>
</body>
</html>
Now we will see this page every time a resource not found error (404) is raised.
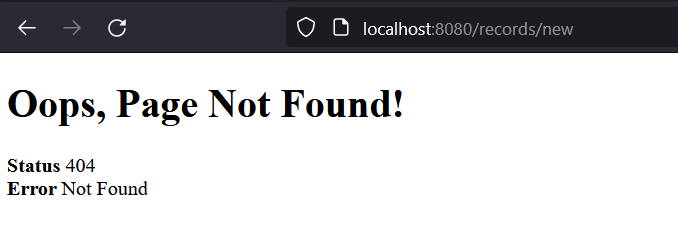
4. Conclusion
This Spring MVC tutorial discussed the default error pages served by a Spring boot application, how to customize those views, and how to change the error page views to even more customized versions.
Happy Learning !!
Comments