In this tutorial, we will learn the Java WeakHashMap and weak references with examples. Also, learn the differences between WeakHashMap and HashMap.
1. Introduction to Java WeakHashMap
The WeakHashMap class (present in java.util package) is a HashTable-based implementation of Map Interface and is present since Java version 1.2. It has almost same features as HashMap including the constructors, methods, performance, and internal implementation.
The primary difference between HashMap and WeakHashMap is that latter has weak keys. An entry in a WeakHashMap will automatically be removed by the garbage collector when the key does not have any strong or soft references. Each key object in a WeakHashMap is stored indirectly as the referent of a weak reference.
Note that the value objects in a WeakHashMap are held by ordinary strong references. So the value objects must not strongly refer to their own keys, either directly or indirectly, since that will prevent the keys from being discarded.
In Java Collections, WeakHashMap class has been declared as follows:
public class WeakHashMap<K, V> extends AbstractMap<K, V> implements Map<K, V>
As shown above, it implements Map
interface and extends AbstractMap
class.
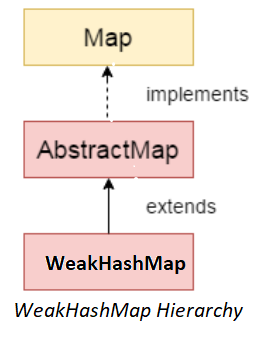
2. Working with WeakHashMap
2.1 Creating WeakHashMap
We can create WeakHashMap by making use of the following constructors:
WeakHashMap()
: Used to create an empty map with the default initial capacity of 16 and default load factor 0.75.WeakHashMap(int initialCapacity)
: Used to create an empty map with the given initial capacity and the default load factor 0.75.WeakHashMap(int initialCapacity, float loadFactor)
: Used to create an empty map with the given initial capacity and the given load factor.WeakHashMap(Map m)
: Used to create a new WeakHashMap with the same entries as the specified map.
WeakHashMap<String, String> map = new WeakHashMap<>();
WeakHashMap<String, String> map = new WeakHashMap<>(16);
WeakHashMap<String, String> map = new WeakHashMap<>(16, 8);
Map<String, String> map = new HashMap<String, String>() {{
put("key1", "value1");
put("key2", "value2");
}};
WeakHashMap<String, String> map = new WeakHashMap<>(map);
2.2 WeakHashMap Methods
Some of the important methods present in WeakHashMap class are:
Object put(key, value)
: Inserts a key-value pair into the map.Object get(key)
: returns the value for the specified key in the map.boolean containsKey(key)
: returnstrue
orfalse
based on whether the specified key is found in the map or not.boolean containsValue(value)
: Similar to containsKey() method, it looks for the specified value instead of key.Set keySet()
: returns the Set of all keys stored in the map.Set entrySet()
: returns the Set of all mappings stored in the map.Value remove(Object key)
: removes the key-value pair for the specified key.int size()
: returns the size of the map which is equal to the number of key-value pairs stored in the map.
2.3 WeakHashMap Example
Let’s quickly cover an example of how to create a WeakHashMap and how we can use the methods described above.
//Creating WeakHashMap
WeakHashMap<Integer, String> map = new WeakHashMap<>();
//Adding values to map using put()
map.put(1, "A");
map.put(2, "B");
map.put(3, "C");
System.out.println(map);
//Getting a value from the map
String value = map.get(2);
System.out.println(value);
//Checking if a key or value present in the map
System.out.println(map.containsKey(3));
System.out.println(map.containsValue("Z"));
//Removing an entry
map.remove(3);
System.out.println(map);
//Finding map size
System.out.println(map.size());
//Iterating over the map
for(Map.Entry<Integer, String> entry : map.entrySet())
{
System.out.println(entry.getKey() + " :: " + entry.getValue());
}
3. Difference Between HashMap and WeakHashMap
In Java, object references can be of many types. Let’s discuss them before digging further into the concept of weak keys in WeakHashMap.
3.1. Strong References, Soft References and Weak References
Strong references are the references we create in a normal program. These are simple variable assignments. In given example, the variable game has a strong reference to a String object with value ‘cricket‘.
Any object having a live strong reference is not eligible for garbage collection.
String game = "cricket";
Soft references is declared explicitly. An object, having a soft reference, won’t be garbage collected until the JVM is about to crash with OutOfMemoryError. JVM will make necessary efforts before reclaiming the memory held by softly referenced objects.
After we make the strong reference null, game object is eligible for GC but will be collected only when JVM absolutely needs memory.
// game is having a strong reference
String game = "cricket";
// Wrapping a strong reference into a soft reference
SoftReference<String> softGame = new SoftReference<>(game);
game = null; //Now it is eligible for GC
Weak references are also created explicitly and are garbage collected eagerly when a GC cycle occurs. GC won’t wait until it needs memory in case of weak references.
In the following example, when we make the strong reference null, the game object can be garbage collected in the next GC cycle, as there is no other strong reference pointing to it.
// strong reference
String game = "cricket";
// Wrapping a strong reference in weak reference
WeakReference<String> softGame = new WeakReference<>(game);
// Making the strong reference as null
game = null; //GC can reclaim it any time
3.2. Garbage Collection Behavior in WeakHashMap
As mentioned earlier, WeakHashMap store the keys as weak references. So when GC is invoked, its keys are eligible for garbage collection.
In following example, we are storing two entries in the map. And we are making one key as null. After the GC runs, we should have only one entry in the map.
Map<MapKey, String> map = new WeakHashMap<>();
MapKey key1 = new MapKey("1");
MapKey key2 = new MapKey("2");
map.put(key1, "1");
map.put(key2, "2");
System.out.println(map);
key1 = null; //Making it GC eligible
System.gc();
Thread.sleep(10000);
System.out.println(map);
{MapKey{key='2'}=2, MapKey{key='1'}=1}
{MapKey{key='2'}=2}
It is possible to put a null key or a null value in the WeakHashMap. The garbage collector only removes the entry from the map for which the key (i.e. object) reference is null. On the other hand, garbage collector won’t remove a key with null value from the WeakHashMap.
4. WeakHashMap Usecases
We can use WeakHapMap in creating simple caches or registry like data-structures where we want to put the objects in the form of key-value pair. When an entry is removed from the such cache then it will no longer be needed in the application.
This clears our cache from time to time by removing unused objects from the memory so that the memory will not fill up with anonymous objects that we are not using anymore in our application.
5. Converting HashMap to WeakHashMap
To create a WeakHashMap from a HashMap, we can use its constructor new WeakHashMap(hashmap)
.
// Creating HashMap
HashMap<Integer, String> hashMap = new HashMap<>();
hashMap.put(1, "A");
hashMap.put(2, "B");
System.out.println("HashMap : " + hashMap);
// Creating WeakHashMap from a HashMap
WeakHashMap<Integer, String> weakHashMap = new WeakHashMap<>(hashMap);
System.out.println("WeakHashMap : " + weakHashMap);
HashMap : {1=A, 2=B}
WeakHashMap : {2=B, 1=A}
6. Conclusion
That’s all about WeakHashMap in Java. We have seen what it is and how it is different from HashMap.
We have also covered practical examples involving both HashMap & WeakHashMap and how they behave differently w.r.t garbage collection. Then we have seen various reference types that we have along with the practical use case for it at the end.
Happy Learning !!
Comments