Java Hashtable class is an implementation of hash table data structure. It is very much similar to HashMap in Java, with most significant difference that Hashtable is synchronized while HashMap is not.
In this Hashtable tutorial, we will learn it’s internals, constructors, methods, use-cases and other important points.
Table of Contents 1. How Hashtable Works? 2. Hashtable Features 3. Hashtable Constructors 4. Hashtable Methods 5. Hashtable Example 6. Hashtable Performance 6. Hashtable vs HashMap 8. Conclusion
1. How Hashtable Works?
Hashtable internally contains buckets in which it stores the key/value pairs. The Hashtable uses the key’s hashcode to determine to which bucket the key/value pair should map.
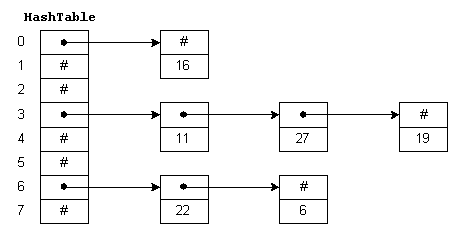
The function to get bucket location from Key’s hashcode is called hash function. In theory, a hash function is a function which when given a key, generates an address in the table. A hash function always returns a number for an object. Two equal objects will always have the same number while two unequal objects might not always have different numbers.
When we put objects into a hashtable, it is possible that different objects (by the equals() method) might have the same hashcode. This is called a collision. To resolve collisions, hashtable uses an array of lists. The pairs mapped to a single bucket (array index) are stored in a list and list reference is stored in array index.
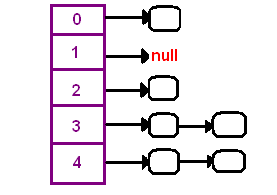
1.1. Hashtable Declaration
The Hashtable class is declared as following in Java. It extends Dictionary class and implements Map, Cloneable
and Serializable
interfaces. Here 'K'
is the type of keys and 'V'
is the type of mapped values to keys.
public class Hashtable<K,V> extends Dictionary<K,V> implements Map<K,V>, Cloneable, java.io.Serializable { //implementation }
2. Hashtable Features
The important things to learn about Java Hashtable class are:
- It is similar to HashMap, but it is synchronized while HashMap is not synchronized.
- It does not accept
null
key or value. - It does not accept duplicate keys.
- It stores key-value pairs in hash table data structure which internally maintains an array of list. Each list may be referred as a bucket. In case of collisions, pairs are stored in this list.
- Enumerator in Hashtable is not fail-fast.
3. Hashtable Constructors
Hashtable class has four constructors.
- Hashtable(): It is the default constructor. It constructs a new, empty hashtable with a default initial capacity (11) and load factor (0.75).
- Hashtable(int size): It constructs a new, empty hashtable of specified initial size.
- Hashtable(int size, float fillRatio): It constructs a new, empty hashtable of specified initial size and fill ratio.
- Hashtable(Map m): It constructs a hashtable that is initialized with the key-value pairs in specified map.
Please note that initial capacity refers to number of buckets in hashtable. An optimal number of buckets is required to store key-value pairs with minimum collisions (to improve performance) and efficient memory utilization.
The fill ratio determines how full hashtable can be before it’s capacity is increased. It’s Value lie between 0.0 to 1.0.
4. Hashtable Methods
The methods in Hashtable class are very similar to HashMap. Take a look.
- void clear() : It is used to remove all pairs in the hashtable.
- boolean contains(Object value) : It returns
true
if specified value exist within the hash table for any pair, else returnfalse
. Note that this method is identical in functionality tocontainsValue()
function. - boolean containsValue(Object value) : It returns
true
if specified value exist within the hash table for any pair, else returnfalse
. - boolean containsKey(Object key) : It returns
true
if specified key exist within the hash table for any pair, else returnfalse
. - boolean isEmpty() : It returns
true
if the hashtable is empty; returnsfalse
if it contains at least one key. - void rehash() : It is used to increase the size of the hash table and rehashes all of its keys.
- Object get(Object key) : It returns the value to which the specified key is mapped. Returns null if no such key is found.
- Object put(Object key, Object value) : It maps the specified
key
to the specifiedvalue
in this hashtable. Neither the key nor the value can benull
. - Object remove(Object key) : It removes the key (and its corresponding value) from hashtable.
- int size() : It returns the number of entries in the hash table.
5. Hashtable Example
Let’s see a example for how to use Hashtable in java programs.
import java.util.Hashtable; import java.util.Iterator; public class HashtableExample { public static void main(String[] args) { //1. Create Hashtable Hashtable<Integer, String> hashtable = new Hashtable<>(); //2. Add mappings to hashtable hashtable.put(1, "A"); hashtable.put(2, "B" ); hashtable.put(3, "C"); System.out.println(hashtable); //3. Get a mapping by key String value = hashtable.get(1); //A System.out.println(value); //4. Remove a mapping hashtable.remove(3); //3 is deleted //5. Iterate over mappings Iterator<Integer> itr = hashtable.keySet().iterator(); while(itr.hasNext()) { Integer key = itr.next(); String mappedValue = hashtable.get(key); System.out.println("Key: " + key + ", Value: " + mappedValue); } } }
Program Output.
{3=C, 2=B, 1=A} A Key: 2, Value: B Key: 1, Value: A
6. Hashtable Performance
Performance wise HashMap performs in O(log(n)) in comparion to O(n) in Hashtable for most common operations such as get(), put(), contains() etc.
The naive approach to thread-safety in Hashtable (“synchronizing every method”) makes it very much worse for threaded applications. We are better off externally synchronizing a HashMap. A well thought design will perform much better than Hashtable.
Hashtable is obsolete. Best is to use ConcurrentHashMap class which provide much higher degree of concurrency.
7. Hashtable vs HashMap
Let’s quickly list down the differences between a hashmap and hashtable in Java.
- HashMap is non synchronized. Hashtable is synchronized.
- HashMap allows one null key and multiple null values. Hashtable doesn’t allow any null key or value.
- HashMap is fast. Hashtable is slow due to added synchronization.
- HashMap is traversed by Iterator. Hashtable is traversed by Enumerator and Iterator.
- Iterator in HashMap is fail-fast. Enumerator in Hashtable is not fail-fast.
- HashMap inherits AbstractMap class. Hashtable inherits Dictionary class.
8. Conclusion
In this tutorial, we learned about Java Hashtable class, it’s constructors, methods, real life usecases and compared their performances. We also learned how a hastable is different from hashmap in Java.
Do not use Hashtable in your new applications. Use HashMap if you do not need councurrency. In concurrent environment, prefer to use ConcurrentHashMap.
Drop me your questions in comments.
Happy Learning !!
Reference:
Comments