If you are using RESTEasy 3.x.y which has been support for JAX-RS 2.0, and you are trying to write RESTEasy client then you can face this exception. Full stack trace might look like this:
Exception in thread "main" java.lang.RuntimeException: java.lang.ClassNotFoundException: org.glassfish.jersey.client.JerseyClientBuilder at javax.ws.rs.client.ClientBuilder.newBuilder(ClientBuilder.java:103) at test.jaxrs2.Demo_JAXRS_2_Example.main(Demo_JAXRS_2_Example.java:14) Caused by: java.lang.ClassNotFoundException: org.glassfish.jersey.client.JerseyClientBuilder at java.net.URLClassLoader$1.run(Unknown Source) at java.security.AccessController.doPrivileged(Native Method) at java.net.URLClassLoader.findClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClassInternal(Unknown Source) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Unknown Source) at javax.ws.rs.client.FactoryFinder.newInstance(FactoryFinder.java:113) at javax.ws.rs.client.FactoryFinder.find(FactoryFinder.java:206) at javax.ws.rs.client.ClientBuilder.newBuilder(ClientBuilder.java:86) ... 1 more
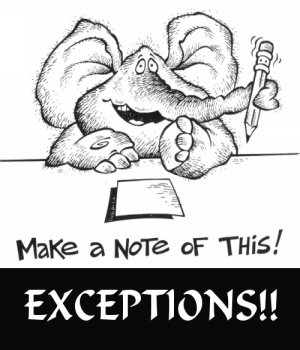
Root cause
Probably you are following trying to wrire the client code as follows:
Client client = ClientBuilder.newBuilder().build(); WebTarget target = client.target("http://localhost:8080/RESTEasyApplication/user-management/users"); Response response = target.request().get(); String users = response.readEntity(String.class);
JAX-RS relies on the Service Provider convention. So, you will need to do multiple things to get it work. Follow below thread for more insight:
https://stackoverflow.com/questions/17366266/jax-rs-2-0-change-default-implementation
Solution
Import RESTEasy client with specific version number as of your resteasy.
<dependency> <groupId>org.jboss.resteasy</groupId> <artifactId>resteasy-client</artifactId> <version>3.0.2.Final</version> </dependency>
This will provide necessary classes to build client for sending RESTful requests.
If still you get any error then use following classes :
ResteasyClient client = new ResteasyClientBuilder().build(); ResteasyWebTarget target = client.target("http://localhost:8080/RESTEasyApplication/user-management/users"); Response response = target.request().get(); String value = response.readEntity(String.class); System.out.println(value); response.close();
It will solve the error.
Happy Learning !!
Comments