Learn how to create a List from an array using Arrays.asList(array) and new ArrayList(Arrays.asList(array). The List created using these methods differs in various ways. Let’s explore some of the main differences between the two.
1. Introduction
Arrays is a utility class present in java.util package and has been there since Java version 1.2. It provides various utility methods to work with an array of objects. Arrays.asList() is one of the methods to create a List from the given array. It won’t create an independent List object, rather it returns a List view i.e. the List created is of fixed size, and no elements can be added or removed from it.
public static List asList(Object[] arr)
ArrayList class present in java.util package extends AbstractList class and implements List Interface and has been there since Java version 1.2. We can create a List from an existing array by using one of the constructors of this class. It creates an independent List instance, which is not of fixed size and changes as per the requirement.
ArrayList list = new ArrayList(Arrays.asList(array))
2. Difference between Arrays.asList(array) & new ArrayList(Arrays.asList(array))
2.1. The Returned List
Arrays.asList(array) creates a List wrapper on the underlying array and returns a List of type java.util.Arrays.ArrayList
which is different from java.util.ArrayList. It gives a list view for an array, and no elements are physically copied to the created List.
// Defining String Array
String[] stringArray = {"a", "b", "c"};
// Creating List using Arrays.asList() method
List<String> listofStrings = Arrays.asList(stringArray);
// Printing class name
System.out.println(listofStrings.getClass().getCanonicalName()); // java.util.Arrays.ArrayList
List created using new ArrayList(Arrays.asList(array)) is of type java.util.ArrayList
class. We are passing a list wrapper to the ArrayList constructor and the constructor physically copies all the elements from it and creates a new independent ArrayList object.
// Defining String Array
String[] stringArray = {"a", "b", "c"};
// Creating List using new ArrayList()
List<String> listofStrings = new ArrayList<>(Arrays.asList(stringArray));
// Printing class name
System.out.println(listofStrings.getClass().getCanonicalName()); // java.util.ArrayList
2.2. Adding and Removing Elements
Arrays.asList(array) creates a List of fixed size, as arrays are fixed in length, and this method just creates a list wrapper on the underlying array, so the created List also follows the rule of fixed size.
We can’t add or remove the elements to & from this list. If we try to do any such operation that will alter the list size, then the list will throw UnsupportedOperationException.
Note: We cannot change the list size created using Arrays.asList() method, but we can replace the value of an existing element with a new value in this List as replace operation won’t alter the list size.
// Creating List using Arrays.asList() method
List<String> listofStrings = Arrays.asList(stringArray);
// Printing list
System.out.println(listofStrings); // [a, b, c]
// Adding element to list
listofStrings.add(“d”); // Raises UnsupportedOperationException
// Replacing element in list
listofStrings.set(0, “z”);
// Printing list
System.out.println(listofStrings); // [z, b, c]
ArrayList(Arrays.asList(array)) creates an independent List that is not of fixed size; hence, we can add/remove and modify the elements of this list.
// Creating List using new ArrayList()
List<String> listofStrings = new ArrayList<>(Arrays.asList(stringArray));
// Printing list
System.out.println(listofStrings); // [a, b, c]
// Adding element to list
listofStrings.add(“d”);
// Printing list
System.out.println(listofStrings); // [a, b, c, d]
// Replacing element in list
listofStrings.set(0, “z”);
// Printing list
System.out.println(listofStrings); // [z, b, c, d]
2.3. Internal Reference for Underlying Array and List
Both the List and the underlying array point to the same object in the heap memory area, in case a List is created using Arrays.asList(array). We don’t have 2 separate objects in the memory for the input array and the created List.
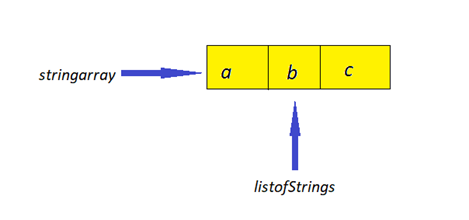
Using new ArrayList(Arrays.asList(array)), we end up having 2 separate objects in the heap memory area, one for the input array and the other for the created List.
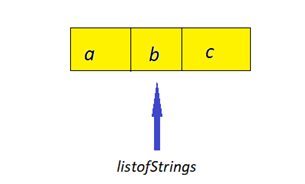
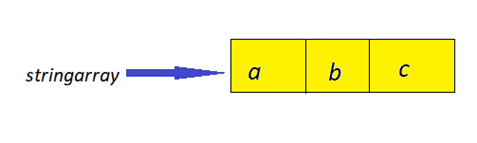
2.4. Changes in Underlying Array on Changing the List Items
As stated above, in the case of Arrays.asList(array), both the input array and the created List point to the same object in the memory. Hence, by using list reference, if we perform any change, the same change will be reflected in the underlying array and vice-versa.
// Creating List using Arrays.asList() method
List<String> listofStrings = Arrays.asList(stringArray);
// Printing underlying array & list
System.out.println(Arrays.toString(stringArray)); // [a, b, c]
System.out.println(listofStrings); // [a, b, c]
// Replacing element in list
listsofStrings.set(0, “z”);
// Printing underlying array & list again
System.out.println(Arrays.toString(stringArray)); // [z, b, c]
System.out.println(listofStrings); // [z, b, c]
For new ArrayList(Arrays.asList(array)), we have 2 separate objects for the underlying array and the created List; modifying one won’t affect the other in any way.
// Creating List new ArrayList()
List<String> listofStrings = new ArrayList<>(Arrays.asList(stringArray));
// Printing underlying array & list
System.out.println(Arrays.toString(stringArray)); // [a, b, c]
System.out.println(listofStrings); // [a, b, c]
// Replacing element in list
listsofStrings.set(0, “z”);
// Printing underlying array & list again
System.out.println(Arrays.toString(stringArray)); // [a, b, c]
System.out.println(listofStrings); // [z, b, c]
3. When to Use?
If our requirement is to create an unmodifiable List from an array i.e. we need a list from which we can frequently read the data, and we won’t need any kind of insertion/deletion operation on that list, then we can use Arrays.asList() method.
On the other hand, if our requirement is to create an independent modifiable list from an array, from which we can read the data and also we can modify the list by adding/removing the elements, then we can use new ArrayList(Array.asList()) instead.
Performance-wise, Arrays.asList() performs better than new ArrayList(Array.asList()). The latter takes O(n)
time complexity to create a List from an array as it internally uses System.arrayCopy()
method to copy the elements from the array to the list.
While Arrays.asList() performs the operation in O(1)
time complexity as no coping of data needed in this case from an input array to the list.
4. Converting Arrays.asList() to ArrayList
Let’s see the various ways by which we can convert a List obtained using Arrays.asList() to a new ArrayList().
4.1. Using ArrayList Constructor
We can use new ArrayList() constructor of ArrayList class to get an independent List object.
ArrayList<String> list = new ArrayList<>(Arrays.asList(stringArray));
4.2. Using Traditional For-Each Loop
We can use for-each loop to iterate over the list generated using Arrays.asList() and add each element into the ArrayList.
// Creating list using Arrays.asList() method
List<String> listofStrings = Arrays.asList(stringArray);
// Creating ArrayList
ArrayList<String> list = new ArrayList<>();
// Loop over list
for(String str : listofStrings){
// Adding str in ArrayList
list.add(str);
}
// Printing ArrayList reference
System.out.println(list); // [a, b, c]
// Modifying the created ArrayList
list.add(“d”);
list.add(“e”);
// Printing ArrayList reference again
System.out.println(list); // [a, b, c, d, e]
5. Conclusion
We have learnt to convert an array into a List by using 2 ways – Arrays.asList() & new ArrayList(Arrays.asList()). We have also seen the main differences between the two and how they work internally along with practical examples and use-cases.
Happy Learning !!
Comments