In this example, we will create a jersey RESTful API web application using jersey-quickstart-webapp maven archetype in eclipse.
Table of Contents Install remote archetypes in eclipse Create new maven project using jersey-quickstart-webapp Generated Files Run The Application
Install remote archetypes in eclipse
The very first step before creating actual maven quick-start application, is to install quick-start definitions in eclipse. It’s very simple process and I have already covered this information in previous tutorial.
Read More: How to Import Maven Remote Archetype Catalogs in Eclipse
Create new maven project using jersey-quickstart-webapp
Now let’s create new maven project.
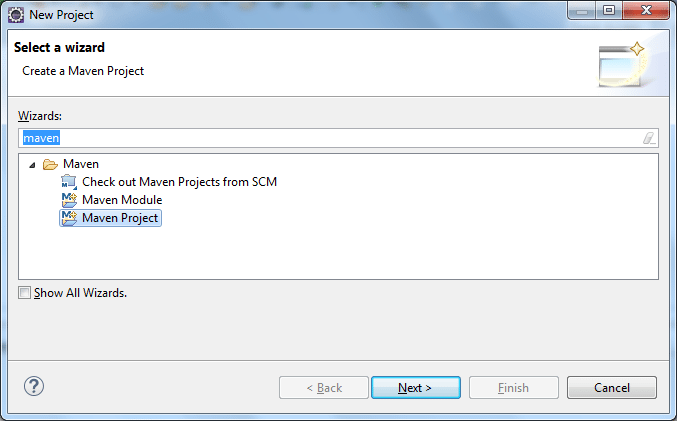
Select default/other workspace.
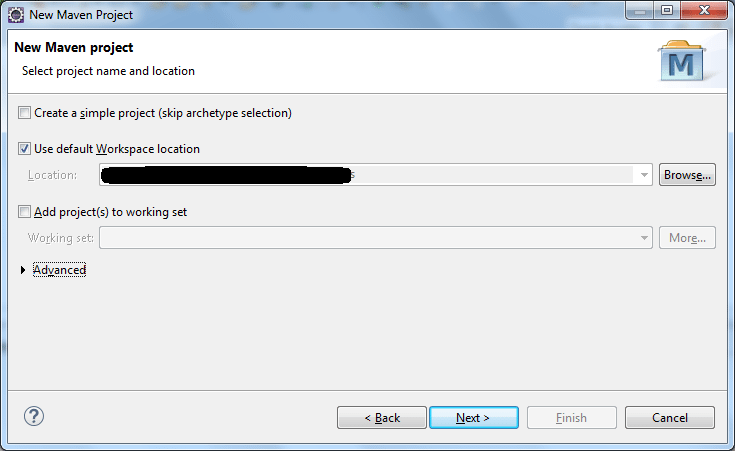
Select jersey-quickstart-webapp
version.
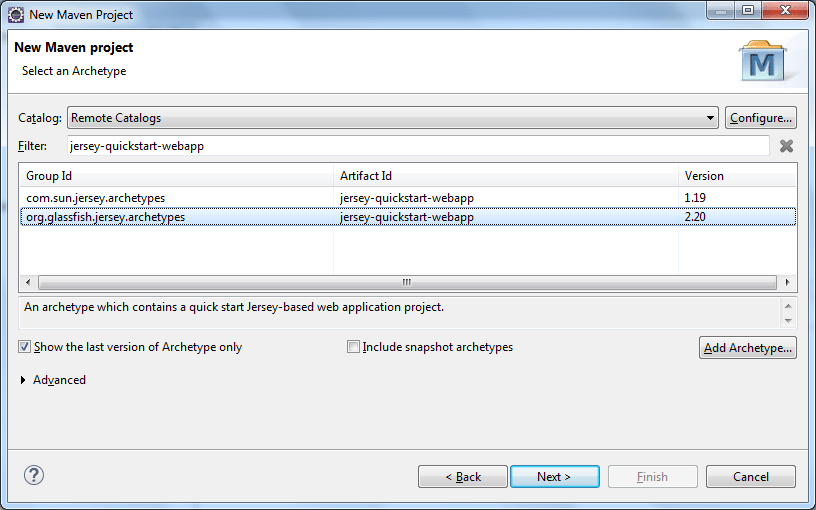
Fill artifact Id and Group Id.
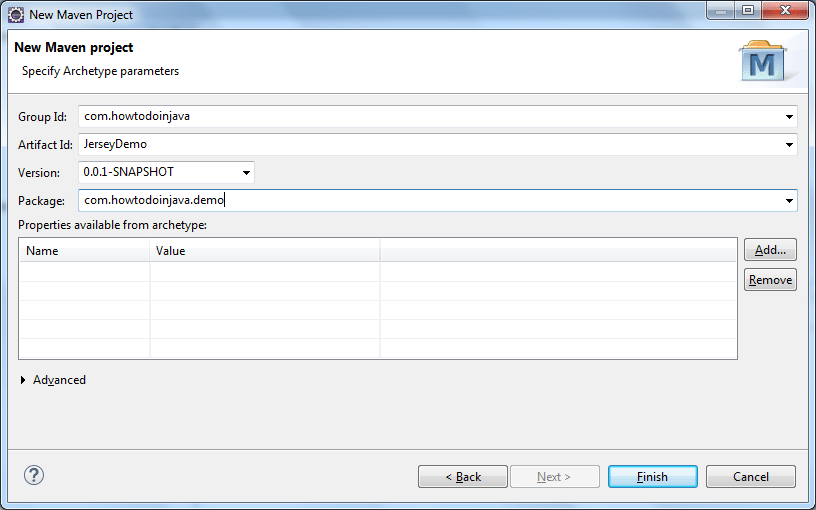
Click ‘OK’ to create this project. Project will be created in workspace.
Generated Files
Let’s look at generated files.
Folder Structure
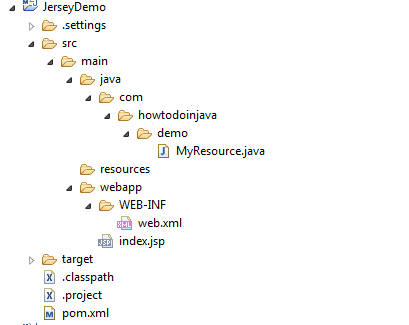
web.xml
<?xml version="1.0" encoding="UTF-8"?> <!-- This web.xml file is not required when using Servlet 3.0 container, see implementation details https://github.com/jax-rs --> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://www.oracle.com/java/technologies/; version="2.5"> <servlet> <servlet-name>Jersey Web Application</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>jersey.config.server.provider.packages</param-name> <param-value>com.howtodoinjava.demo</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>Jersey Web Application</servlet-name> <url-pattern>/webapi/*</url-pattern> </servlet-mapping> </web-app>
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.howtodoinjava.demo</groupId> <artifactId>JerseyDemo</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>JerseyDemo</name> <build> <finalName>JerseyDemo</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.5.1</version> <inherited>true</inherited> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build> <dependencyManagement> <dependencies> <dependency> <groupId>org.glassfish.jersey</groupId> <artifactId>jersey-bom</artifactId> <version>${jersey.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <dependencies> <dependency> <groupId>org.glassfish.jersey.containers</groupId> <artifactId>jersey-container-servlet-core</artifactId> <!-- use the following artifactId if you don't need servlet 2.x compatibility --> <!-- artifactId>jersey-container-servlet</artifactId --> </dependency> <!-- uncomment this to get JSON support <dependency> <groupId>org.glassfish.jersey.media</groupId> <artifactId>jersey-media-moxy</artifactId> </dependency> --> </dependencies> <properties> <jersey.version>2.20</jersey.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> </project>
MyResource.java
package com.howtodoinjava.demo; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; /** * Root resource (exposed at "myresource" path) */ @Path("myresource") public class MyResource { /** * Method handling HTTP GET requests. The returned object will be sent * to the client as "text/plain" media type. * * @return String that will be returned as a text/plain response. */ @GET @Produces(MediaType.TEXT_PLAIN) public String getIt() { return "Got it!"; } }
Run the Application
For hello world usage, you don’t need to do anything in this project. Everything is configured. Just deploy the application.
Hit URL : http://localhost:8080/JerseyDemo/webapi/myresource
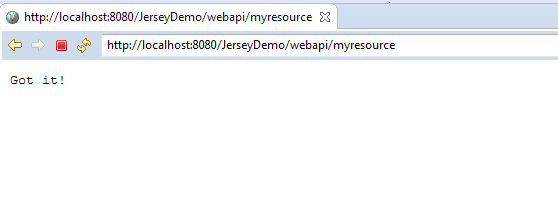
Drop me your questions in comments section.
Happy Learning !!
Comments