In this Java AIML tutorial, we will learn to create a simple chatbot program in Java. A Chatbot is an application designed to simulate conversation with human users, especially over the Internet. Internally it uses any NLP (Natural Language Processing) system to interpret the human interactions and reply back with meaningful information.
In 2024 (and beyond), a better approach is to use a pre-trained LLM model and feed it domain-specific knowledgebase using RAG.
Using AIML library to create rule-based chatbots is an older technology, and using a more advanced NLP solution, such as OpenAI GPT, Google Dialogflow, Rasa or IBM Watson, will be a better decision.
AIML (Artificial Intelligence Markup Language) is an XML dialect for creating natural language software agents. It contains the basic rules which Natural Language Understanding (NLU) unit uses internally. It can be thought of as the heart of the engine. The more rules we add in AIML – the more intelligent our Chatbot will be.
It’s important to know the difference between NLP and NLU.
- NLP refers to all systems that work together to handle end-to-end interactions between machines and humans in the preferred language of the human. In other words, NLP lets people and machines talk to each other “naturally”.
- NLU is actually a subset of the wider world of NLP. It helps in parsing unstructured inputs e.g. mispronunciations, swapped words, contractions, colloquialisms, and other quirks.
1. Prerequisites
- Reference AIML Implementation – To get started, we shall use an already working reference application. There is one such java based implementation called program-ab hosted on google-code repository. Download the
program-ab
latest distribution from maven code repository. - Eclipse and Maven – for coding and development.
2. Java AIML Chatbot Example
Follow these simple steps for building our first Chatbot application.
2.1. Download Unzip the program-ab Distribution
We need to first unzip the program-ab
distribution to a convenient folder. We will need to take Ab.jar
and existing AIML rules from it.
2.2. Create eclipse project
Create an eclipse maven project to start the development. It involves pretty standard steps so I am assuming that you will be able to do it yourself.
2.3. Import AIML library
After we have created the maven project to start the development, let us choose packaging as jar and maven coordinate as your choice and import to eclipse.
Now create a folder lib
in the base folder and copy the Ab.jar
from the program-ab distribution to this folder.
2.4. Add AIML to Classpath
To add AIML to classpath, add Ab.jar
to deployment assembly in eclipse. Alternatively, you can install this jar into your local maven repository and then use it.
To install locally, place the jar in any folder and provide that location in the systemPath tag. Now, add below AIML maven dependency to pom.xml. Now build the maven project by command mvn clean install
.
<dependencies>
<dependency>
<artifactId>com.google</artifactId>
<groupId>Ab</groupId>
<version>0.0.4.3</version>
<scope>system</scope>
<systemPath>${basedir}/src/main/resources/lib/Ab.jar</systemPath>
</dependency>
</dependencies>
2.5. Copy Default AIML Rules
Copy the bots
folder from program-a
b directory into the resources
folder of your maven project. This folder contains default AIML sets that we will use initially. Later we will see how we can add our custom rules into our Chatbot.
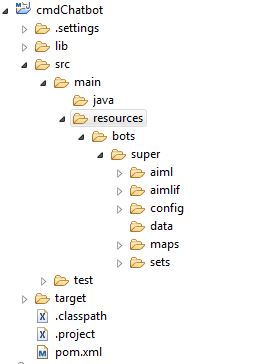
2.6. Create Hello World Chatbot Program
Now create a simple java program i.e. Chatbot.java
. It’s main()
method will invoke the chat program which will run on the command prompt.
The basic structure of this program will be to introduce an infinite loop and in each loop take a user input from the command prompt and then we will ask program-ab API to give the answer to the input provided by the user.
More details about the program-ab API interaction are mentioned in the wiki link.
package com.howtodoinjava.demo.chatbot;
import java.io.File;
import org.alicebot.ab.Bot;
import org.alicebot.ab.Chat;
import org.alicebot.ab.History;
import org.alicebot.ab.MagicBooleans;
import org.alicebot.ab.MagicStrings;
import org.alicebot.ab.utils.IOUtils;
public class Chatbot {
private static final boolean TRACE_MODE = false;
static String botName = "super";
public static void main(String[] args) {
try {
String resourcesPath = getResourcesPath();
System.out.println(resourcesPath);
MagicBooleans.trace_mode = TRACE_MODE;
Bot bot = new Bot("super", resourcesPath);
Chat chatSession = new Chat(bot);
bot.brain.nodeStats();
String textLine = "";
while (true) {
System.out.print("Human : ");
textLine = IOUtils.readInputTextLine();
if ((textLine == null) || (textLine.length() < 1))
textLine = MagicStrings.null_input;
if (textLine.equals("q")) {
System.exit(0);
} else if (textLine.equals("wq")) {
bot.writeQuit();
System.exit(0);
} else {
String request = textLine;
if (MagicBooleans.trace_mode)
System.out.println(
"STATE=" + request + ":THAT=" + ((History) chatSession.thatHistory.get(0)).get(0)
+ ":TOPIC=" + chatSession.predicates.get("topic"));
String response = chatSession.multisentenceRespond(request);
while (response.contains("<"))
response = response.replace("<", "<");
while (response.contains(">"))
response = response.replace(">", ">");
System.out.println("Robot : " + response);
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
private static String getResourcesPath() {
File currDir = new File(".");
String path = currDir.getAbsolutePath();
path = path.substring(0, path.length() - 2);
System.out.println(path);
String resourcesPath = path + File.separator + "src" + File.separator + "main" + File.separator + "resources";
return resourcesPath;
}
}
2.7. Test Chatbot Interactions
Now our program is ready and we can now start a conversation with chat. To do that just run the program as a java application. To exit, you shall type q or wq
.
Human : Hi
Robot : Hi! It's delightful to see you.
Human : How are you
Robot : Feeling really joyful today.
Human : My name is sajal
Robot : Glad to see you, Sajal
Human : what is my name
Robot : Sajal
Human : tell me my name
Robot : Sajal
Like this, you can do basic talk with the chatbot.
3. Add Custom Patterns in Chatbot
3.1. Adding Custom Patterns
Now we can add our custom patterns in the bot to add more intelligence to the interactions. To do that create a new .aiml
file in the aiml
directory of your bot (src\main\resources\bots\super\aiml
).
Add as many categories in that file as you wish. Here category refers to the human questions and the template refers to the chat bot’s response. I have created a file called a-custom-entry.aiml
and added three questions as below.
<?xml version="1.0" encoding="UTF-8"?>
<aiml>
<category>
<pattern>WHAT IS JAVA</pattern>
<template>Java is a programming language.</template>
</category>
<category>
<pattern>WHAT IS CHAT BOT</pattern>
<template>Chatbot is a computer program designed to simulate conversation with human users, especially over the Internet.</template>
</category>
<category>
<pattern>WHAT IS INDIA</pattern>
<template>wonderful country.</template>
</category>
</aiml>
Now we can ask both these three questions as well.
Once our custom aiml file is ready, we need to do generate corresponding entries for other folders parallel to aiml
. To do it, we need to invoke the bot.writeAIMLFiles()
.
I have created another java program called AddAiml.java
for this purpose. Once you are done with the aiml editing, just run this program once before starting the bot. It will add these custom entries to the bot’s brain.
package com.howtodoinjava.demo.chatbot;
import java.io.File;
import org.alicebot.ab.Bot;
import org.alicebot.ab.MagicBooleans;
public class AddAiml {
private static final boolean TRACE_MODE = false;
static String botName = "super";
public static void main(String[] args) {
try {
String resourcesPath = getResourcesPath();
System.out.println(resourcesPath);
MagicBooleans.trace_mode = TRACE_MODE;
Bot bot = new Bot("super", resourcesPath);
bot.writeAIMLFiles();
} catch (Exception e) {
e.printStackTrace();
}
}
private static String getResourcesPath() {
File currDir = new File(".");
String path = currDir.getAbsolutePath();
path = path.substring(0, path.length() - 2);
System.out.println(path);
String resourcesPath = path + File.separator + "src"
+ File.separator + "main" + File.separator + "resources";
return resourcesPath;
}
}
3.2. Testing Custom Patterns
After running the AddAiml
, once you have added the new entries into the AIML, run the chatbot program again and ask the new questions. It should give a proper response.
In my case, here is the output.
Human : What is java Robot : Java is a programming language. Human : what is chat bot Robot : Chatbot is a computer program designed to simulate conversation with human users, especially over the Internet. Human : what is india Robot : wonderful country.
4. Conclusion
In this AIML Java tutorial, we have learned to create a simple command-line based chatbot program with program-ab reference application. Next time, when you have any such requirement, you can think of AIML based chatbot. It is capable of doing moderate stuff easily.
To enhance your knowledge, you may try to –
- Play with AIML and create more interesting conversations. AIML has many tags which you can use, it also give some option to configure Sraix to invoke external REST based web services.
- Add your own custom placeholder in the template section and parse that from the AIML response and do more based on your identifier in the response, like You can able to invoke your own web service to get some more information.
- Convert this command line chat program to web based chat, I am currently working on that and will publish a followup post on that.
- You can add your custom rule engine on top of your AIML response to do more.
AIML can do lots of things, but nowadays much more sophisticated techniques are available especially in the Machine Learning space, try to learn those as well.
Happy Learning !!
Comments