In this Java tutorial, we will learn the difference between decimal and hexadecimal numbers, and how to convert a decimal number to a hexadecimal number and back using built-in Java APIs and custom methods.
1. Difference between Decimal and Hexadecimal Numbers
1.1. Difference
A decimal number uses 10 symbols (i.e. base 10) ranging from [0-9] to represent a number, while a hexadecimal number uses 16 symbols (i.e. base 16) ranging from [0-9, A-F].
The radix (or base) of a decimal number is 10 i.e. all digits of a decimal number are represented in terms of power of 10. And the radix of a hexadecimal number is 16 i.e. all digits of a hexadecimal number are represented in terms of power of 16.

1.2. Mathematical Conversion
As the hexadecimal numbers are represented in terms of the power of 16, we can divide a given decimal number by 16 and consider the reminder to get the corresponding hexadecimal number. Let’s understand this by an example.
Let’s walk through step by step to Convert 269 (a decimal number) to hexadecimal
- Take 269 as the dividend and 16 as the divisor
- Divide the dividend with the divisor and store the remainder
- Divide the quotient obtained from the above step by 16 and store the remainder.
- Repeat the third step until the dividend becomes zero.
- The final hexadecimal number will be the reverse of the stored remainder.
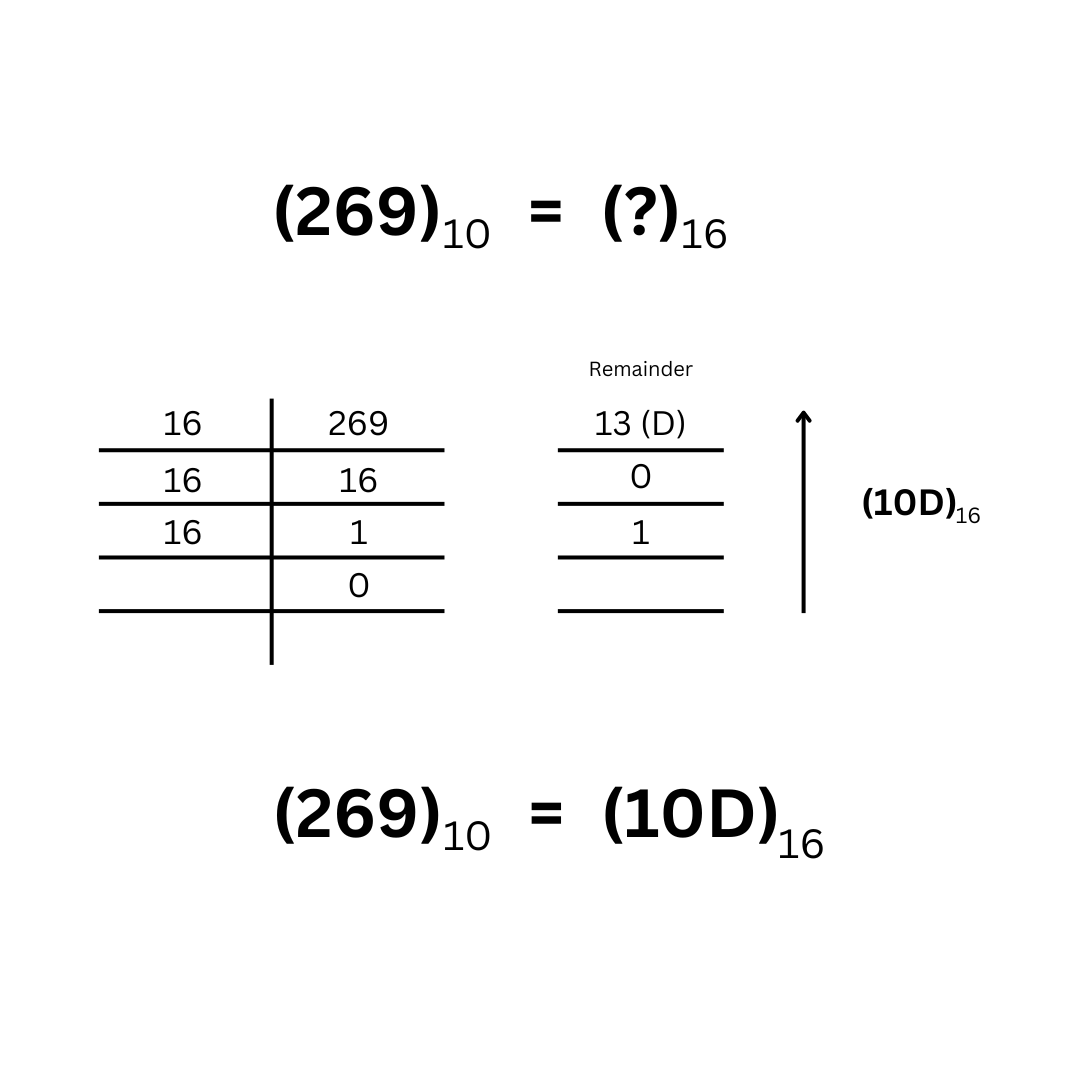
2. Representing Numbers of Different Bases in Java
In Java, all Number data types (int, long, float, double) always represent the values in decimal format. It’s never possible that an int/float can hold any number in binary, octal, or hexadecimal format.
We can assign binary, octal, or hexadecimal values to an int/long using predefined prefixes.
int hexNum = 0X10D; // '0X' or '0x' prefix to represent hexadecimal number
int octNumber = 01560; // '0' prefix to represent octal number
Still, at last, it will be converted to decimal, and printing that variable will print the value in the decimal form only.
System.out.println(hexNum); // 269
System.out.println(octNumber); // 880
Hence, we can never get a hexadecimal, octal, or binary representation of any number in an int or other decimal types datatypes, so we need to represent hex/octal/binary numbers in String format only.
3. Converting a Decimal Number to Hexadecimal
let us see a few simple and easy methods to convert a given decimal to hexadecimal.
3.1. Using Number Classes
All Java number classes provide built-in methods for performing the conversion from decimal to equivalent hex number. Let us list down these methods:
For Integer and Long classes:
- Integer.toString(num, radix);
- Integer.toHexString(num);
- Long.toString(num, radix);
- Long.toHexString(num);
- BigInteger.toString(Radix);
For Float and Double classes:
- Float.toHexString(num);
- Double.toHexString(num);
In toString() methods, we pass the radix (16 for hexadecimal) along with the number to convert.
int number = 269;
String hex = Integer.toString(number, 16); // '10d'
The toHexString() method directly converts the given number into hexadecimal form.
double number = 333.44d;
String hex = Double.toHexString(number); // '0x1.4d70a3d70a3d7p8'
The BigInteger class also provides a toString(radix) method for the same purpose.
BigInteger number = new BigInteger("269");
String hex = number.toString(16); // '10d'
3.2. Using String.format()
The String class has an overloaded format() method that accepts a format specifier. The format to represent hexadecimal numbers is %x . This method can be used to convert a decimal integer number into a hexadecimal string.
int number = 269;
String hex = String.format("%x", number); // '10d'
3.3. Using a Custom Method
We can also create a custom method and code for the algorithm discussed in the first section of this article.
- Perform the long division of the decimal number.
- Take the remainder of the division.
- Get the hexadecimal token according to the remainder.
- Prepend the token into the result.
- Repeat these steps until the result of the division is 0.
public static String convertToHex(int number) {
if (number <= 0) {
return "0";
}
int remainder;
StringBuilder result = new StringBuilder();
String tokens = "0123456789ABCDEF";
while (number > 0) {
remainder = number % 16;
result.insert(0, tokens.charAt(remainder));
number = number / 16;
}
return result.toString();
}
Now we can use this method for the decimal-to-hex conversion in Java.
String hex = convertToHex(269);
System.out.println(hex); // '10D'
4. Converting a Hexadecimal Number to Decimal
4.1. Using Number Classes
Converting from hexadecimal strings to Java Number types is easy if converting to Integer and Long types. There is direct API support for such conversion:
- Integer.parseInt(hex, radix);
- Integer.valueOf(hex, radix);
- Integer.decode(hex);
- Long.parseLong(hex, radix);
- Long.valueOf(hex, radix);
- Long.decode(hex);
- new BigInteger(hex, radix);
The following example converts a hexadecimal to Integer type using the defined methods. The same can be done for hexadecimal to Long conversion.
String hex = "10D";
int decimal = Integer.parseInt(hex,16);
//or
int decimal = Integer.valueOf(hex,16);
//or
int decimal = Integer.decode("Ox" + hex);
The BigInteger class also provides a constructor new BigInteger(hex, radix) for the same purpose.
BigInteger decimal = new BigInteger("10d",16);
System.out.println(decimal); // 269
Converting a hexadecimal to floating-point numbers (float and double) is not straightforward. We need to perform the following steps:
- Parse the hexadecimal string to an integer
- Use
Float.intBitsToFloat()
orDouble.longBitsToDouble()
to interpret the integer as a float or double value.
String myString = "BF800000";
Long i = Long.parseLong(myString, 16);
Float f = Float.intBitsToFloat(i.intValue());
//converted float value
System.out.println(f);
//Convert the float back to hex and verify
System.out.println(Integer.toHexString(Float.floatToIntBits(f)));
Program output:
-1.0
bf800000
4.2. Using a Custom Method
To convert a hexadecimal string into a decimal integer using the raw method, we will use the below simple algorithm.
- Find the decimal number of the corresponding hexadecimal token
- First, multiply the variable in which we are going to add this decimal token by 16 and then add the decimal number.
- Repeat these steps till the last token of the hexadecimal string.
public static int convertHexToDecimal(String hex) {
String tokens = "0123456789ABCDEF";
hex = hex.toUpperCase();
int result = 0;
for (int i = 0; i < hex.length(); i++) {
int n = tokens.indexOf(hex.charAt(i));
result = result * 16 + n;
}
return result;
}
Let us test the method.
System.out.println(convertHexToDecimal("10D")); //269
5. Conclusion
In this Java data types tutorial, we learned about the difference between decimal and hexadecimal numbers, and the conversion of decimal to hexadecimal numbers using the APIs and custom methods.
Happy Learning !!
Comments