Learn how to set classpath in Java either as an environment variable and pass as the command-line argument. During runtime of any Java application, the CLASSPATH is a parameter that tells the JVM where to look for classes and packages.
- The default value of the classpath is “
.
”(dot)
, meaning that only the current directory is searched for dependencies. - Specifying either the
CLASSPATH
environment variable or the-cp
command line switch overrides this value. - The order in which you specify multiple classpath entries is important. The Java interpreter will look for classes in the directories in the order they appear in the classpath variable.
Java Classpath separators are OS specific.
Windows –;
[Semicolon]
Linux/Unix –:
[Colon]
CLASSPATH
as Environment Variable
1. Setting When you have set the location of jar files that are always required during the application runtime, then it’s probably best to add them in the machine’s environment variable 'CLASSPATH'
.
During application runtime, application class loader will always scan the jar files and classes at specified paths in this variable.
To set CLASSPATH
environment variable, find the location of user environment variables in your machine and add all paths where Jar files are stored. Use the separator between different two folders, jar files or classes.
You can find the user environment variables window by –
- From the desktop, right-click the Computer icon.
- Choose Properties from the context menu.
- Click the Advanced system settings link.
- Click Environment Variables. In the section System Variables, find the
CLASSPATH
environment variable and select it. Click Edit. If theCLASSPATH
environment variable does not exist, clickNew
. - Add all folders separated with a separator. Click OK. Close all remaining windows by clicking OK.
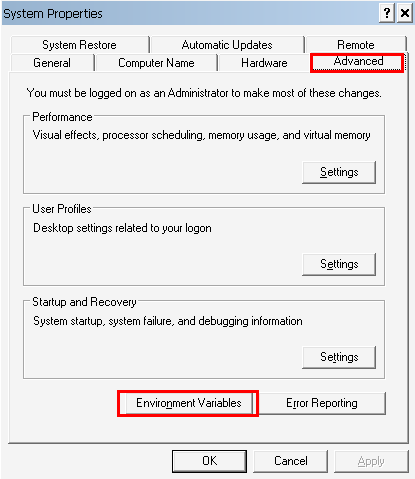
If you are creating CLASSPATH for the first time, you need to specify the name for the variable name in Windows 10. Use ‘.’ (dot) to denote the current directory.
2. Setting CLASSPATH from Command Line
Use -classpath
argument to set classpath from command prompt/console. Use the following command to set the classpath for different requirements.
Let’s say we have a folder named dependency
where JAR files and other classes are placed.
2.1. Add a Single Jar in CLASSPATH
Below syntax examples will add single jar file in classpath.
//WINDOWS
$ set CLASSPATH=.;C:\dependency\framework.jar
//Linux/Unix
$ export CLASSPATH=.:/dependency/framework.jar
2.2. Add Multiple Jars in CLASSPATH
Below syntax examples will add more than one jar file in classpath. To do so, simply use the delimiter for your operating system (either ;
or :
) as a separator between the locations specified for the CLASSPATH.
To add all JAR files present in a directory, use wildcard character ('*'
).
//WINDOWS
$ set CLASSPATH=C:\dependency\framework.jar;C:\location\otherFramework.jar
$ set CLASSPATH=C:\dependency\framework.jar;C:\location\*.jar
//Linux/Unix
$ export CLASSPATH=/dependency/framework.jar:/location/otherFramework.jar
$ export CLASSPATH=/dependency/framework.jar:/location/*.jar
2.3. Add Multiple Classes in CLASSPATH
Many times, you may need to add individual classes in classpath as well. To do so, simply add the folder where classfile is present. e.g. let’s say there are five .class
files are present in location
folder which you want to include in classpath.
//WINDOWS
$ set CLASSPATH=C:\dependency\*;C:\location
//Linux/Unix
$ export CLASSPATH=/dependency/*:/location
As a best practice, always organize all JAR files and application classes inside one root folder. This may be the workspace for the application.
Please note that subdirectories contained within the CLASSPATH would not be loaded. In order to load files that are contained within subdirectories, those directories and/or files must be explicitly listed in the CLASSPATH.
2.4. Clear the CLASSPATH
If your CLASSPATH environment variable was set to a value that is not correct, then you can unset CLASSPATH by specifying an empty value to it.
set CLASSPATH=
3. Executing Programs with ‘-classpath’ or ‘-cp’ Option
Apart from setting the classpath to the environment variable, you can pass an additional classpath to Java runtime while launching the application using –classpath
option or –cp
option.
Use the .
(dot) to include the current path in the classpath where the .class
file has been generated.
$ javac –classpath C:\dependency\framework.jar MyApp.Java
$ java –classpath .;C:\dependency\framework.jar MyApp
CLASSPATH
Value
4. Print Current Anytime you wish to verify all path entries in CLASSPATH
variable, you can verify using the echo command.
//Windows c:/> echo %CLASSPATH% //Linux/Unix $ echo $CLASSPATH
If CLASSPATH is not set you will get a CLASSPATH: Undefined variable error (Solaris or Linux) console or simply %CLASSPATH% printed in the windows command prompt.
Happy Learning !!
Read More:
Java – How to set classpath in Windows 7, 8, 10
Java – Set classpath from the command line
Comments