Inside a Java program, we can write a special text that will be ignored by the Java compiler — known as the comment. Comments allow us to exclude code from the compilation process (disable it) or clarify a piece of code to yourself or other developers.
Learn everything about Java comments, types of Java comments, Javadoc tool, the performance impact of comments and best practices to follow.
1. Why should we write comments?
The comments, as the name suggests, are notes we write between the programs for various reasons. For example, you may write comments to –
- Write information or explanation about the variable, method, class, or any statement.
- Write text to be available in Java docs.
- Hide program code for a specific time, etc.
Given code is an example of Java comments, and how to use them.
/**
* Contains method to greet users by their name and location.
*
* @author Lokesh Gupta
*/
public class Main {
/**
* Launches the application
*
* @param args - Application startup arguments
*/
public static void main(String[] args) {
getMessage("Lokesh", "India");
}
/**
* Returns welcome message for a customer by customer name and location
*
* @param name - Name of the visitor
* @param region - Location
* @return - Welcome message
*/
public static String getMessage (String name, String region)
{
StringBuilder builder = new StringBuilder();
builder.append("Hello ");
builder.append(name);
builder.append(", Welcome to ");
builder.append(region);
builder.append(" !!");
return builder.toString();
}
}
2. Types of Java Comments
There are 3 types of comments in Java.
2.1. Single Line Comment
Use single-line comments when the comment can be written in a single line only. These comments are written over Java statements to clarify what they are doing.
//Initialize the counter variable to 0
int counter = 0;
2.2. Multi-Line Comment
Use multi-line comments when you need to add information in source code that exceeds more than one line. Multi-line comments are used mostly above code blocks with complex logic that cannot be written in a single line.
/*
* This function returns a variable which shall be used as a counter for any loop.
* Counter variable is of integer type and should not be reset during execution.
*/
public int getCounter() {
//
}
2.3. Java Documentation Comment
The documentation comments are used when you want to expose information to be picked up by the javadoc
tool for creating the HTML documentation for classes by reading the source code. This is the information you see in editors (e.g. eclipse) when using the autocomplete feature. These comments are pit above classes, interfaces, and method definitions.
- Documentation comments start with
/**
and end with*/
. By convention, each line of a doc comment begins with a*
, as shown in the following example, but this is optional. Any leading spacing and the*
on each line are ignored. - Within the doc comment, lines beginning with
@
are interpreted as special instructions for the documentation generator, giving it information about the source code. - You can use javadoc annotations inside these comments e.g.
@param
and@return
.
/**
* This function returns a variable which shall be used as a counter for any loop.
* Counter variable is of integer type and should not be reset during execution.
*
* @param seed - initial value of the counter
* @return counter value
*/
public int getCounter(int seed) {
//
}
Doc comments can appear above class, method, and variable definitions, but some tags may not apply to all of these. For example, the @exception
tag can only be applied to methods.
Tag | Description | Applies to |
---|---|---|
@see | Associated class name | Class, method, or variable |
@code | Source code content | Class, method, or variable |
@link | Associated URL | Class, method, or variable |
@author | Authjor name | Class |
@version | Version number | Class |
@param | Parameter name and description | Method |
@return | Return type and description | Method |
@exception | Exception name and description | Method |
@deprecated | Declares an item to be obsolete | Class, method, or variable |
@since | Notes API version when item was added | Variable |
Documentation comments are an integral part of programming and should not be skipped.
3. Comment Shortcuts
In Eclipse IDE, simply typing “/** [Enter]” before a public method or class will automatically generate in all necessary @param
, @author
and @return
attributes.
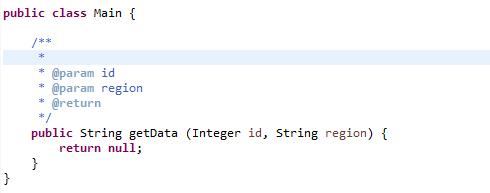
4. Javadoc Utility
Javadoc utility is bundled with JDK distributions. It converts them into standardized, nicely formatted, easy-to-read web pages. It generates API documentation from documentation comments.
4.1. Run javadoc from the command prompt
First, make sure javadoc
the utility is in your PATH. If not then add JDK/bin folder to PATH variable.
To generate Java docs, execute the utility with two arguments. First is location of generated Java docs, and second is Java source files. In our case, I executed this command from location where Main.java
is.
$ javadoc -d C:/temp Main.java
It generated these Java docs HTML files.
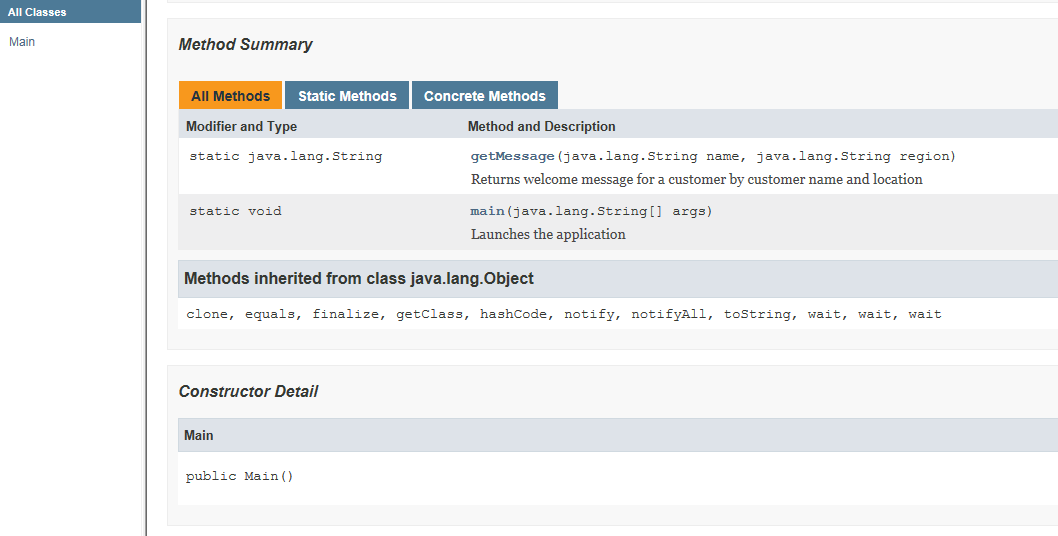
4.2. Run javadoc from Eclipse
You can generate the Java documentation from Eclipse IDE as well. Follow these simple steps-
- In the Package Explorer, right-click the desired project/package.
- Select
Export.../Javadoc
and clickNext
.
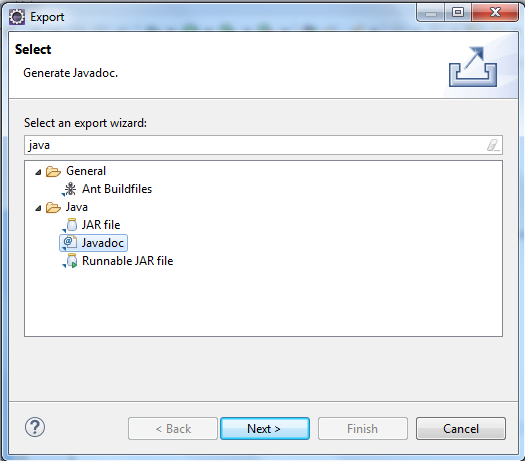
- By default, the entire source code will be selected. Verify and change your selections.
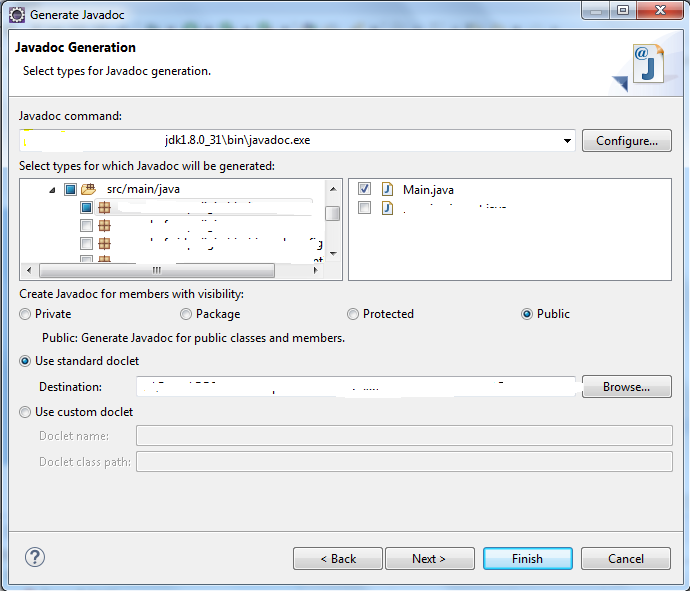
- You may select “
Private
” for the visibility level to be generated. This will generate all possible Javadocs, even for private methods. - Select the “
standard doclet
” which is the destination folder for your documentation. - Click
Next
. - Enter a meaningful
Document title
and clickFinish
.
If you follow all above steps correctly, you will have generated Java docs file similar to what we saw in command prompt option.
5. Performance Impact of Java Comments
Implementation comments in Java code are only there for humans to read. The Java comments are statements that are not compiled by the compiler, so they are not included into compiled bytecode (.class
file).
And that’s why Java comments have no impact on application performance as well.
6. Java Comments Best Practices
Follow these best practices to have proper comments in your application source code.
- Do not use unnecessary comments in the source code. If your code needs more than a normal explanation, then possibly re-factor your code.
- Keep comments indentation uniform and match for best readability.
- Comments are for humans so use simple language to explain.
- Always add documentation comments in your source code.
Happy Learning !!