The Math class in Java consists of methods performing common mathematical operations. Two important methods within this class for handling absolute values are Math.abs() and Math.absExact(). This Java tutorial explores what are absolute values, overflow, and underflow problems of abs() method and how to absExact() solve them.
1. What is an Absolute Value?
The absolute value of a real number, often denoted by |x|, is the non-negative value of x without regard to its sign. In other words, it is the distance of the number from zero on the number line.
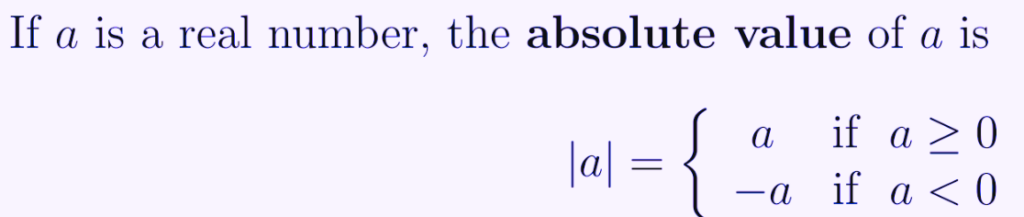
Here are a few examples to illustrate the concept:
- ∣5∣=5 because 5 is a positive number.
- ∣−8∣=8 because the absolute value of -8 is 8.
- ∣0∣=0 because the absolute value of 0 is 0.
The absolute values are commonly used for computing/expressing distances, for example, distance from the sea level. The distance from sea level is always a positive number whether a scuba diver is diving under the sea or a mountain climber climbing on the mountain.
2. Java Math.abs() Function
The Math.abs() is used for obtaining the absolute value of a numeric value or expression. It accepts the arguments of type int, long, float, and double.
public static double abs(double a)
public static float abs(float a)
public static int abs(int a)
public static long abs(long a)
For example, we can use the abs() as follows:
double doubleValue = -10.56;
float floatValue = -7.8f;
int intValue = -15;
long longValue = -123456789L;
System.out.println(STR."Absolute value of double: \{Math.abs(doubleValue)}");
System.out.println(STR."Absolute value of float: \{Math.abs(floatValue)}");
System.out.println(STR."Absolute value of int: \{Math.abs(intValue)}");
System.out.println(STR."Absolute value of long: \{Math.abs(longValue)}");
The program output:
Absolute value of double: 10.56
Absolute value of float: 7.8
Absolute value of int: 15
Absolute value of long: 123456789
3. The Overflow/Underflow Problem
While Math.abs() itself does not directly lead to overflow or underflow issues, understanding the behavior of this function is crucial when working with large numeric values, as it may indirectly contribute to such problems.
Consider the following program
int intMinValue = Integer.MIN_VALUE;
long longMinValue = Long.MIN_VALUE;
System.out.println(STR."Absolute value of int: \{Math.abs(intMinValue)}");
System.out.println(STR."Absolute value of long: \{Math.abs(longMinValue)}");
The program output:
Absolute value of int: -2147483648
Absolute value of long: -9223372036854775808
This is not good. Absolute values should never be negative values. In these cases, the absolute value is larger than the MAX_VALUE thus causing the underflow issue.
If we are not careful of such boundary cases, we can get unexpected results in the programs.
4. Preventing Overflow/Underflow using Math.absExact()
Starting with JDK 15, the Math class was enriched with two absExact() methods. There is one for int and one for long datatypes. If the mathematical absolute result is prone to overflowing or underflowing the int
or long
boundaries, these methods throw an ArithmeticException instead of returning a misleading result.
Let us re-run the previous program with absExact() method.
System.out.println(STR."Absolute value of int: \{Math.absExact(intMinValue)}");
Exception in thread "main" java.lang.ArithmeticException: Overflow to represent absolute value of Integer.MIN_VALUE
at java.base/java.lang.Math.absExact(Math.java:1903)
at com.howtodoinjava.core.basic.MathAbsoluteExamples.main(MathAbsoluteExamples.java:25)
For non-boundary values, the Math.abs() and Math.absExact() work similarly.
If you are still on the Java version before 15, you can write the check for the boundary case yourself as follows:
public static int absExact(int a) {
if (a == Integer.MIN_VALUE)
throw new ArithmeticException(
"Overflow to represent absolute value of Integer.MIN_VALUE");
else
return abs(a);
}
5. Conclusion
Since Java 15 onwards, it is recommended to use Math.absExact() everywhere you are calculating the absolute value of a number. This will prevent overflow and underflow issues automatically. Before Java 15, add the check for boundary cases yourself.
If you need to work with very large numbers or require arbitrary precision, consider using specialized classes like BigInteger
or BigDecimal
that can handle such scenarios without overflow or underflow.
Happy Learning !!
Comments