Java concurrency allows multiple threads to run together within a single program. However, writing multi-threaded code can be challenging, as concurrent access to shared resources can lead to synchronization issues such as race conditions and deadlocks.
In this post, we will explore how local variables and method parameters in Java are thread-safe and why this is important in a multi-threaded environment.
1. Java Memory Model
In Java, local variables, objects, and methods are all stored in different areas of memory depending on their scope and lifecycle. We can learn more about how these are stored in Java Memory Model tutorial.
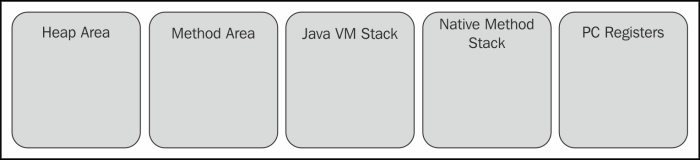
In runtime, Each of the JVM threads has a private stack created at the same time when the thread is created. The stack frames are used to store data including local variables such as primitives and object references, method parameters, and partial results of computations to perform dynamic linking, return values for methods, and dispatch exceptions.
Note that the Java Virtual Machine uses local variables to pass parameters on method invocation.
2. Why are Local Variables Thread-Safe?
In Java, local variables are inherently thread-safe because they are stored on the stack memory, which is a unique memory space allocated to each thread of execution.
When a thread invokes a method, it creates a new stack frame that contains the local variables for that method. This means each thread has its own copy of the local variables and can access and modify them independently of other threads.
For example, consider a simple Java program that uses local variables in a multi-threaded environment:
public class MyRunnable implements Runnable {
public void run() {
int x = 0;
while (x < 10) {
System.out.println(Thread.currentThread().getName() + ": " + x);
x++;
}
}
public static void main(String[] args) {
MyRunnable r = new MyRunnable();
Thread t1 = new Thread(r);
Thread t2 = new Thread(r);
t1.start();
t2.start();
}
}
In this program, the MyRunnable
class implements the Runnable interface, which allows it to be run in a separate thread of execution. The run()
method contains a local variable x
that is initialized to 0
and incremented by 1
in a loop. The main()
method creates two threads that run the MyRunnable
instance concurrently.
Because each thread has its own stack memory, the local variable x
in the run()
the method is thread-safe. Each thread has its own copy x
and can modify it independently of the other thread. This means that the output of the program is non-deterministic and depends on the order in which the threads execute:
Thread-0: 0
Thread-0: 1
Thread-0: 2
Thread-1: 0
Thread-1: 1
Thread-1: 2
Thread-0: 3
Thread-1: 3
Thread-0: 4
Thread-1: 4
...
...
3. Thread-Safety of Local Variables in Streams
In Java streams, variables used in stream operations are generally thread-safe. This is because streams operate on a data source and return a new stream as the output, without modifying the original data source. Therefore, the state of the original data source is not modified, and the variables used in stream operations do not change.
However, there are some cases where thread safety may be compromised. For example, if the stream operation involves modifying a shared state variable, then thread safety can be compromised. In such cases, it is important to use synchronization or atomic operations to ensure thread safety.
Let’s take an example to illustrate this. Suppose we have a list of integers and we want to perform some operations on them using streams. We have a shared state variable ‘sum‘ that we want to use to keep track of the sum of the integers.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = 0;
sum = numbers.stream()
.mapToInt(Integer::intValue)
.peek(x -> sum += x)
.sum();
In this example, we are using the ‘sum‘ variable inside the peek() method to keep track of the sum of the integers. However, since ‘sum‘ is a shared state variable, this code is not thread-safe. So the question is how to make this code thread-safe.
To make the code thread-safe, you can use synchronization mechanisms such as synchronized blocks or the AtomicInteger class.
3.1. Using synchronized Blocks
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = 0;
synchronized(numbers) {
sum = numbers.stream()
.mapToInt(Integer::intValue)
.peek(x -> {
synchronized(this) {
sum += x;
}
})
.sum();
}
In this modified code, we use two synchronized blocks to ensure that access to the numbers
list and the sum
variable is synchronized between threads.
- The outer synchronized block locks the
numbers
list, which prevents other threads from modifying it while the stream is being processed. - The inner synchronized block locks the
sum
variable, which ensures that only one thread can modify it at a time.
3.2. Using Concurrent Types
Alternatively, we can use the AtomicInteger
class to make the sum
variable thread-safe without using synchronized blocks:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
AtomicInteger sum = new AtomicInteger(0);
sum.addAndGet(numbers.stream()
.mapToInt(Integer::intValue)
.sum());
In this modified code, we use the AtomicInteger
class to make the sum
variable thread-safe. The addAndGet()
method atomically adds the sum of the numbers in the stream to the sum
variable, ensuring that no other threads can modify it at the same time.
Overall, while variables used in streams are generally thread-safe, it is important to be mindful of shared state variables and take necessary precautions to ensure thread safety when working with them.
4. FAQs
Local variables in Java are thread-safe because they are stored on the stack memory, which is private to each thread. This means that each thread has its own copy of the local variables, which eliminates the possibility of concurrent access and modification by other threads.
No, not all variables in Java are thread-safe. Variables that are shared between threads, such as instance variables and static variables, are not thread-safe by default and require synchronization to ensure thread safety.
To ensure thread safety when using shared resources in Java, you can use synchronization mechanisms such as synchronized methods, synchronized blocks, or the java.util.concurrent package. You can also use thread-safe collections such as ConcurrentHashMap or synchronized collections.
Yes, thread safety can be achieved in Java without synchronization by using thread-local variables or immutable objects. However, these approaches may not be suitable for all scenarios and may require a different design or programming approach.
5. Conclusion
In this post, we have explored why local variables in Java are thread-safe and how they are stored in memory. We have also discussed the thread safety of variables used in streams and how to ensure thread safety when using shared resources. By understanding these concepts, you can write multi-threaded Java code that is both efficient and powerful.
Happy Learning !!
Comments