Hibernate was started in 2001 by Gavin King as an alternative to using EJB2-style entity beans. Its mission back then was to simply offer better persistence capabilities than offered by EJB2 by simplifying the complexities and allowing for missing features.
Hibernate used its mapping files and configuration files to achieve its objectives. With the introduction of annotations in the java community with JDK 1.5, Hibernate community started working on Hibernate 3, which had support for annotations. The current version is Hibernate 6.
In this hibernate example with annotations, we will learn more about hibernate and step by step build our first running example application for beginners.
1. What is Hibernate?
Hibernate is an open-source object relational mapping tool for Java. It provides a framework for mapping an object-oriented domain model to a traditional relational database. Hibernate not only takes care of the mapping of Java classes to database tables (and from Java data types to SQL data types) but also provides data query and retrieval facilities and can significantly reduce development time otherwise spent with manual data handling in SQL and JDBC.
The mapping Java classes to database tables is accomplished through the configuration of an XML file or by using Java annotations. There are facilities to arrange one-to-one, one-to-many and many-to-many relationships between classes are provided.
In addition to managing associations between objects, Hibernate can also manage reflexive associations where an object has a one-to-many relationship with other instances of its own type.
2. How does Hibernate Work?
Hibernate doesn’t get in our way; nor does it force us to change the way our objects behave. The objects don’t need to implement any magical interfaces in order to be blessed with the ability to persist. All we have to do to put some metadata in form of annotations telling hibernate how to use them when mapping them with the database. At runtime, hibernate reads these annotations and use this information to build queries to send to some relational database.
There is a simple, intuitive API in Hibernate to perform queries against the objects represented by the database, to change those objects we just interact with them normally in the program, and then tell Hibernate to save the changes. Creating new objects is similarly simple; we just create them in the normal way and tell Hibernate about them using annotations so they can get stored in the database.
3. Relation of Hibernate with JPA
JPA (Java Persistence API) is a specification for persistence providers to implement. Hibernate is one such implementation of JPA specification. We can annotate our classes as much as we would like with JPA annotations, however without an implementation, nothing will happen.
Think of JPA as the guidelines/specification that must be followed or an interface, while Hibernate’s JPA implementation is code that meets the API as defined by JPA and provides the under the hood functionality.
When we use hibernate with JPA we are actually using the Hibernate’s JPA implementation. The benefit of this is that we can swap out hibernates implementation of JPA for another implementation of the JPA specification.
When we use straight hibernate your locking into the implementation because other ORMs may use different methods/configurations and annotations, therefore we cannot just switch over to another ORM.
4. Building Hello World Application
Let us create our step by step hibernate 5 hello world example. In this example, I have created an Employee
class and declared four attributes id
, email
, firstname
and lastname
.
I want the id
attribute should be generated automatically so that the application code does not store a local cache of employee ids.
So far we targeted what we want to make in our first application. Let’s identify the files that need to be created.
- hibernate.cfg.xml -This configuration file will be used to store database connection information and schema level settings.
- EmployeeEntity.java – This class will refer to Java POJOs having the hibernate annotations.
- HibernateUtil.java – This class will have utility methods that will be used for creating SessionFactory and Session objects.
- TestHibernate.java – This class will be used to test our configuration settings and Emplyee entity annotations.
Before moving into code, let us see the project setup and add maven dependencies which need to add to pom.xml to include all compile-time and runtime dependencies.
4.1. Create a maven project
Read create a simple maven project article for detailed steps.
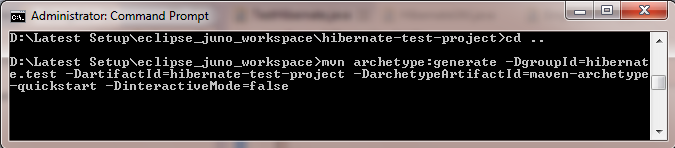
4.2. Add IDE Support (Optional)
This is an optional step. I am using Eclipse in this demo.
$ mvn eclipse:eclipse
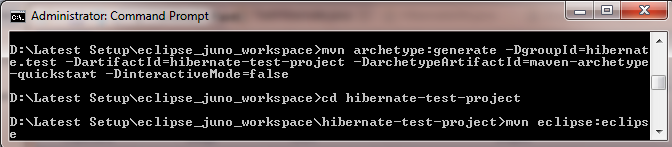
4.3. Import Project into Eclipse
Import the project into eclipse.
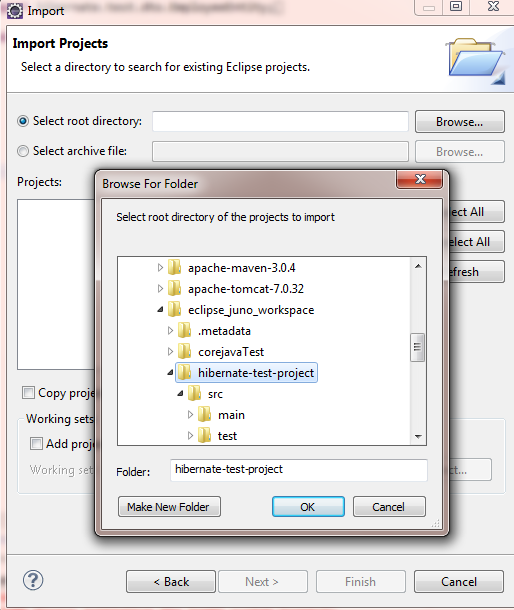
The above steps will create the minimum setup. Now it’s time to add hibernate dependencies.
4.4. Maven Dependencies
At a minimum, we will need hibernate-core dependency. We are using in-memory database H2 for this example. So include com.h2database
dependency as well.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.howtodoinjava</groupId>
<artifactId>hibernate-hello-world</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.3.7.Final</version>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<version>1.4.200</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<sourceDirectory>src/main/java</sourceDirectory>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.5.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
4.5. Hibernate Configuration
Notice the connection parameters use the H2 database-related properties. Also, add the entities to be persisted as part of metadata.
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">org.h2.Driver</property>
<property name="hibernate.connection.url">jdbc:h2:mem:test</property>
<property name="hibernate.connection.username">sa</property>
<property name="hibernate.connection.password"></property>
<property name="hibernate.dialect">org.hibernate.dialect.H2Dialect</property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">create-drop</property>
<mapping class="com.howtodoinjava.hibernate.test.dto.EmployeeEntity"></mapping>
</session-factory>
</hibernate-configuration>
4.6. Entity To Persist
Annotate the entities with JPA annotations.
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.UniqueConstraint;
import org.hibernate.annotations.OptimisticLockType;
@Entity
@org.hibernate.annotations.Entity(optimisticLock = OptimisticLockType.ALL)
@Table(name = "Employee", uniqueConstraints = {
@UniqueConstraint(columnNames = "ID"),
@UniqueConstraint(columnNames = "EMAIL") })
public class EmployeeEntity implements Serializable {
private static final long serialVersionUID = -1798070786993154676L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "ID", unique = true, nullable = false)
private Integer employeeId;
@Column(name = "EMAIL", unique = true, nullable = false, length = 100)
private String email;
@Column(name = "FIRST_NAME", unique = false, nullable = false, length = 100)
private String firstName;
@Column(name = "LAST_NAME", unique = false, nullable = false, length = 100)
private String lastName;
// Accessors and mutators for all four fields
}
Starting Hibernate 6, the default JPA provider packages have been moved from javax.*
to jakarta.*
.
import java.io.Serializable;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.Table;
import jakarta.persistence.UniqueConstraint;
@Entity
@Table(name = "Employee", uniqueConstraints = {
@UniqueConstraint(columnNames = "ID"),
@UniqueConstraint(columnNames = "EMAIL") })
public class EmployeeEntity implements Serializable {
//..
}
5. Creating SessionFactory and Session
Build the SessionFactory using StandardServiceRegistry and Metadata.
import org.hibernate.SessionFactory;
import org.hibernate.boot.Metadata;
import org.hibernate.boot.MetadataSources;
import org.hibernate.boot.registry.StandardServiceRegistry;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
public class HibernateUtil {
private static SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory()
{
try
{
if (sessionFactory == null)
{
StandardServiceRegistry standardRegistry = new StandardServiceRegistryBuilder()
.configure("hibernate.cfg.xml").build();
Metadata metaData = new MetadataSources(standardRegistry)
.getMetadataBuilder()
.build();
sessionFactory = metaData.getSessionFactoryBuilder().build();
}
return sessionFactory;
} catch (Throwable ex) {
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
public static void shutdown() {
getSessionFactory().close();
}
}
6. Demo
import org.hibernate.Session;
import com.howtodoinjava.hibernate.test.dto.EmployeeEntity;
public class TestHibernate {
public static void main(String[] args) {
Session session = HibernateUtil.getSessionFactory().openSession();
session.beginTransaction();
//Add new Employee object
EmployeeEntity emp = new EmployeeEntity();
emp.setEmail("demo-user@mail.com");
emp.setFirstName("demo");
emp.setLastName("user");
session.save(emp);
session.getTransaction().commit();
HibernateUtil.shutdown();
}
}
The above code will create a new table EMPLOYEE
in the database and insert one row in this table. In logs, you can verify the insert statement which got executed.
Hibernate: drop table Employee if exists
Hibernate: create table Employee (ID integer generated by default as identity, EMAIL varchar(100)
not null, FIRST_NAME varchar(100) not null, LAST_NAME varchar(100) not null, primary key (ID))
Hibernate: alter table Employee add constraint UK_ardf0f11mfa6tujs3hflthwdv unique (EMAIL)
Hibernate: insert into Employee (ID, EMAIL, FIRST_NAME, LAST_NAME) values (null, ?, ?, ?)
Hibernate: drop table Employee if exists
If you face a problem in running above hibernate hello world example, drop me a comment and I will be glad to discuss the problem with you.
Happy Learning!!
Comments