In this tutorial, learn to create and consume RSS and Atom feed from spring boot application. You must have seen this in form of text or image buttons on various websites (like our RSS feed) inviting you to “Subscribe via RSS.” RSS is simple syndication API – often referred as Rich Site Summary. RSS revolutionized the way that users interact with online content.
Similar to RSS, Atom is also an XML-based web content and metadata syndication format, and an application-level protocol for publishing and editing Web resources belonging to periodically updated websites. All Atom feeds must be well-formed XML documents with the application/atom+xml
media type.
Table of Contents Overview How it works? Project Structure Create RSS/ATOM Feed Generator Create RSS/ATOM Client Summary
Overview
In this boot example, we will expose two simple endpoints for RSS and ATOM using spring boot APIs and the we will see how to consume those feeds using java client.
Technology Stack
- JDK 1.8, Eclipse, Maven – Development environment
- Spring-boot – Underlying application framework
- ROME library – For publishing feed
How it works?
Basically publishing a RSS or Atom feed with the Spring framework is very easy. In spring framework there are two http message converters (RssChannelHttpMessageConverter and AtomFeedHttpMessageConverter) that can convert responses of spring controller methods into the XML feed format, if the return type is related to any of these feeds.
Both converters depend on the ROME library and the Spring Framework does automatically registers these two converters when it discovers the library on the classpath. All we have to do is adding the ROME library as a dependency to our pom.xml
.
Project Structure
The classes and files created for this demo are given below.
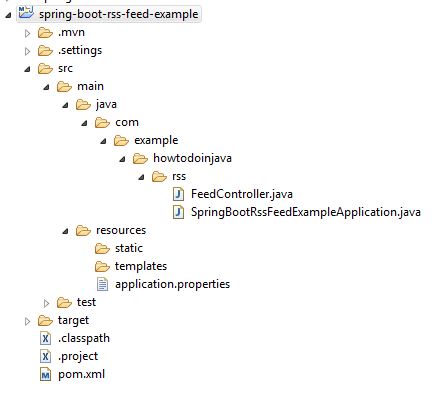
Create RSS / ATOM Feed Generator
Create Spring Boot Project
Start with creating one spring boot project from Spring Initializer site with Web
dependency only. After selecting the dependency and giving the proper maven GAV coordinates, download project in zipped format. Unzip and then import project in eclipse as maven project.
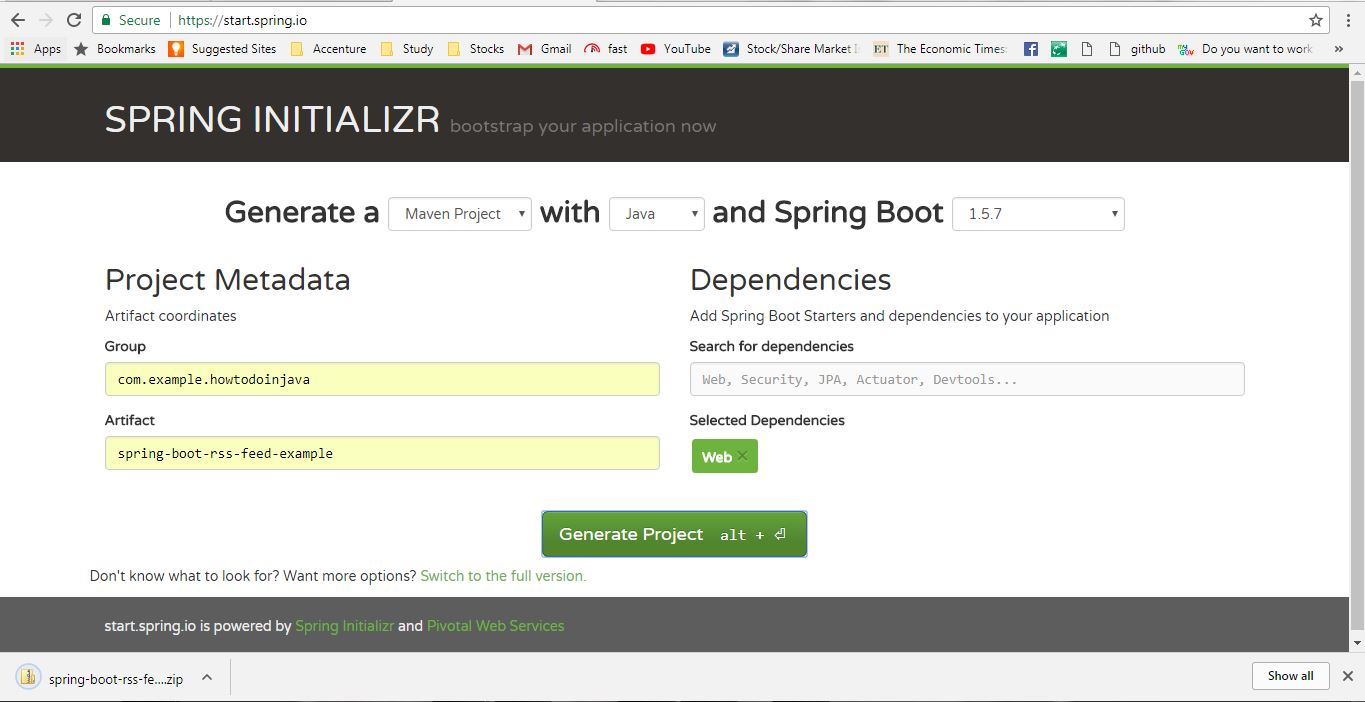
Add ROAM Dependency
Now we need to add ROAM dependency in the pom.xml of the newly created project.
<dependency> <groupId>com.rometools</groupId> <artifactId>rome</artifactId> <version>1.8.0</version> </dependency>
Create Controller
Now add one Spring Controller and add two endpoints /rss
and /atom
to expose the RSS and Atom feed respectively. As we have already mentioned just adding this controller will automatically work for our case as internally spring framework will register two http message converters (RssChannelHttpMessageConverter
and AtomFeedHttpMessageConverter
) which will be registered once we have ROAM dependency in the classpath.
Only thing we need to do this to return proper Feed
type Object from the controller methods. In our case, feed object is of type com.rometools.rome.feed.rss.Channel
for RSS and com.rometools.rome.feed.atom.Feed
for Atom. So after adding the contents of the feed and other details about the channels our controller will look like below.
package com.example.howtodoinjava.rss; import java.util.Collections; import java.util.Date; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; import com.rometools.rome.feed.atom.Category; import com.rometools.rome.feed.atom.Content; import com.rometools.rome.feed.atom.Entry; import com.rometools.rome.feed.atom.Feed; import com.rometools.rome.feed.atom.Link; import com.rometools.rome.feed.atom.Person; import com.rometools.rome.feed.rss.Channel; import com.rometools.rome.feed.rss.Description; import com.rometools.rome.feed.rss.Image; import com.rometools.rome.feed.rss.Item; import com.rometools.rome.feed.synd.SyndPerson; @RestController public class FeedController { @GetMapping(path = "/rss") public Channel rss() { Channel channel = new Channel(); channel.setFeedType("rss_2.0"); channel.setTitle("HowToDoInJava Feed"); channel.setDescription("Different Articles on latest technology"); channel.setLink("https://howtodoinjava.com"); channel.setUri("https://howtodoinjava.com"); channel.setGenerator("In House Programming"); Image image = new Image(); image.setUrl("https://howtodoinjava.com/wp-content/uploads/2015/05/howtodoinjava_logo-55696c1cv1_site_icon-32x32.png"); image.setTitle("HowToDoInJava Feed"); image.setHeight(32); image.setWidth(32); channel.setImage(image); Date postDate = new Date(); channel.setPubDate(postDate); Item item = new Item(); item.setAuthor("Lokesh Gupta"); item.setLink("https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/"); item.setTitle("Spring CORS Configuration Examples"); item.setUri("https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/"); item.setComments("https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/#respond"); com.rometools.rome.feed.rss.Category category = new com.rometools.rome.feed.rss.Category(); category.setValue("CORS"); item.setCategories(Collections.singletonList(category)); Description descr = new Description(); descr.setValue( "CORS helps in serving web content from multiple domains into browsers who usually have the same-origin security policy. In this example, we will learn to enable CORS support in Spring MVC application at method and global level." + "The post <a rel=\"nofollow\" href=\"https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/\">Spring CORS Configuration Examples</a> appeared first on <a rel=\"nofollow\" href=\"https://howtodoinjava.com\">HowToDoInJava</a>."); item.setDescription(descr); item.setPubDate(postDate); channel.setItems(Collections.singletonList(item)); //Like more Entries here about different new topics return channel; } @GetMapping(path = "/atom") public Feed atom() { Feed feed = new Feed(); feed.setFeedType("atom_1.0"); feed.setTitle("HowToDoInJava"); feed.setId("https://howtodoinjava.com"); Content subtitle = new Content(); subtitle.setType("text/plain"); subtitle.setValue("Different Articles on latest technology"); feed.setSubtitle(subtitle); Date postDate = new Date(); feed.setUpdated(postDate); Entry entry = new Entry(); Link link = new Link(); link.setHref("https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/"); entry.setAlternateLinks(Collections.singletonList(link)); SyndPerson author = new Person(); author.setName("Lokesh Gupta"); entry.setAuthors(Collections.singletonList(author)); entry.setCreated(postDate); entry.setPublished(postDate); entry.setUpdated(postDate); entry.setId("https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/"); entry.setTitle("spring-mvc-cors-configuration"); Category category = new Category(); category.setTerm("CORS"); entry.setCategories(Collections.singletonList(category)); Content summary = new Content(); summary.setType("text/plain"); summary.setValue( "CORS helps in serving web content from multiple domains into browsers who usually have the same-origin security policy. In this example, we will learn to enable CORS support in Spring MVC application at method and global level." + "The post <a rel=\"nofollow\" href=\"https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/\">Spring CORS Configuration Examples</a> appeared first on <a rel=\"nofollow\" href=\"https://howtodoinjava.com\">HowToDoInJava</a>."); entry.setSummary(summary); feed.setEntries(Collections.singletonList(entry)); //Like more Entries here about different new topics return feed; } }
Demo
Start the spring boot application, do maven build using mvn clean install
and start the application using java -jar target\spring-boot-rss-feed-example-0.0.1-SNAPSHOT.jar
command. This will bring up one tomcat server in default port 8080
and application will be deployed in it.
Now go to http://localhost:8080/rss and http://localhost:8080/atom from browser and you should see the RSS and Atom feed for the topics that you have added in the controller.
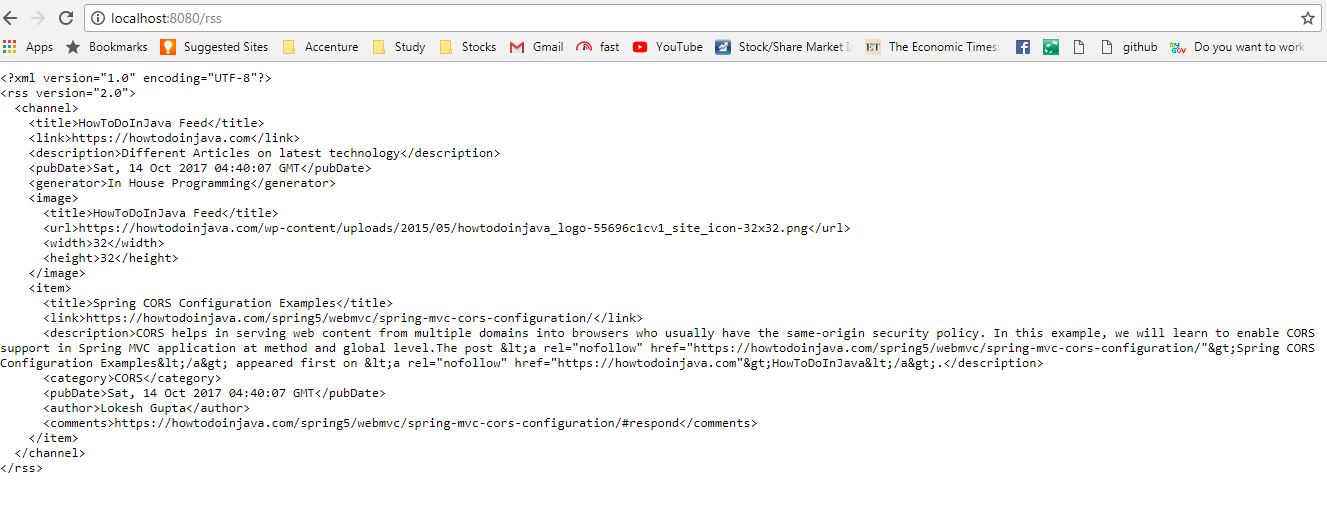
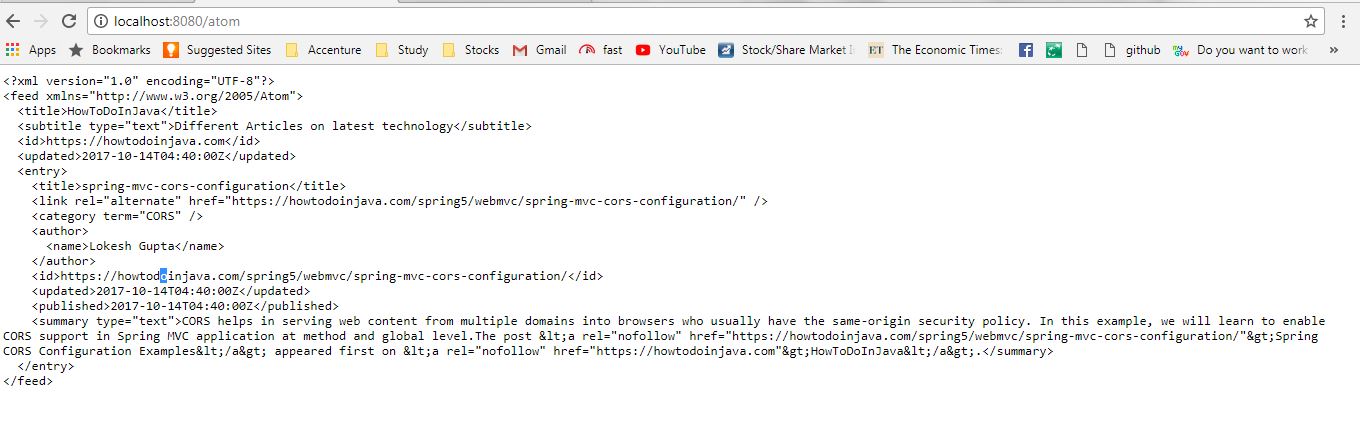
Create RSS Feed Reader
We have already lots of Feed readers available, still if you need to consume this feed programmatically, you can do this by this simple few lines of code using ROAM library.
package com.example.howtodoinjava.rss; import java.net.URL; import com.rometools.rome.feed.synd.SyndEntry; import com.rometools.rome.feed.synd.SyndFeed; import com.rometools.rome.io.SyndFeedInput; import com.rometools.rome.io.XmlReader; public class FeedConsumer { public static void main(String[] args) { try { String url = "http://localhost:8080/rss"; try (XmlReader reader = new XmlReader(new URL(url))) { SyndFeed feed = new SyndFeedInput().build(reader); System.out.println(feed.getTitle()); System.out.println("***********************************"); for (SyndEntry entry : feed.getEntries()) { System.out.println(entry); System.out.println("***********************************"); } System.out.println("Done"); } } catch (Exception e) { e.printStackTrace(); } } }
Here the URL is for RSS feed, similarly the same code will work if you change the URL to Atom feed.
Output
Here is the console output of the feed client.
HowToDoInJava Feed *********************************** SyndEntryImpl.comments=https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/#respond SyndEntryImpl.author=Lokesh Gupta SyndEntryImpl.wireEntry=null SyndEntryImpl.link=https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/ SyndEntryImpl.description.mode=null SyndEntryImpl.description.type=text/html SyndEntryImpl.description.interface=interface com.rometools.rome.feed.synd.SyndContent SyndEntryImpl.description.value=CORS helps in serving web content from multiple domains into browsers who usually have the same-origin security policy. In this example, we will learn to enable CORS support in Spring MVC application at method and global level.The post <a rel="nofollow" href="https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/">Spring CORS Configuration Examples</a> appeared first on <a rel="nofollow" href="https://howtodoinjava.com">HowToDoInJava</a>. SyndEntryImpl.foreignMarkup=[] SyndEntryImpl.source=null SyndEntryImpl.updatedDate=null SyndEntryImpl.title=Spring CORS Configuration Examples SyndEntryImpl.interface=interface com.rometools.rome.feed.synd.SyndEntry SyndEntryImpl.uri=https://howtodoinjava.com/spring5/webmvc/spring-mvc-cors-configuration/ SyndEntryImpl.enclosures=[] SyndEntryImpl.modules[0].date=Sat Oct 14 10:57:12 IST 2017 SyndEntryImpl.modules[0].formats=[] SyndEntryImpl.modules[0].sources=[] SyndEntryImpl.modules[0].rightsList=[] SyndEntryImpl.modules[0].subject=null SyndEntryImpl.modules[0].creators[0]=Lokesh Gupta SyndEntryImpl.modules[0].description=null SyndEntryImpl.modules[0].language=null SyndEntryImpl.modules[0].source=null SyndEntryImpl.modules[0].type=null SyndEntryImpl.modules[0].title=null SyndEntryImpl.modules[0].interface=interface com.rometools.rome.feed.module.DCModule SyndEntryImpl.modules[0].descriptions=[] SyndEntryImpl.modules[0].coverages=[] SyndEntryImpl.modules[0].relation=null SyndEntryImpl.modules[0].contributor=null SyndEntryImpl.modules[0].rights=null SyndEntryImpl.modules[0].publishers=[] SyndEntryImpl.modules[0].coverage=null SyndEntryImpl.modules[0].identifier=null SyndEntryImpl.modules[0].creator=Lokesh Gupta SyndEntryImpl.modules[0].types=[] SyndEntryImpl.modules[0].languages=[] SyndEntryImpl.modules[0].identifiers=[] SyndEntryImpl.modules[0].subjects=[] SyndEntryImpl.modules[0].format=null SyndEntryImpl.modules[0].dates[0]=Sat Oct 14 10:57:12 IST 2017 SyndEntryImpl.modules[0].titles=[] SyndEntryImpl.modules[0].uri=http://purl.org/dc/elements/1.1/ SyndEntryImpl.modules[0].publisher=null SyndEntryImpl.modules[0].contributors=[] SyndEntryImpl.modules[0].relations=[] SyndEntryImpl.contents=[] SyndEntryImpl.links=[] SyndEntryImpl.publishedDate=Sat Oct 14 10:57:12 IST 2017 SyndEntryImpl.contributors=[] SyndEntryImpl.categories[0].taxonomyUri=null SyndEntryImpl.categories[0].name=CORS SyndEntryImpl.categories[0].interface=interface com.rometools.rome.feed.synd.SyndCategory SyndEntryImpl.titleEx.mode=null SyndEntryImpl.titleEx.type=null SyndEntryImpl.titleEx.interface=interface com.rometools.rome.feed.synd.SyndContent SyndEntryImpl.titleEx.value=Spring CORS Configuration Examples SyndEntryImpl.authors=[] *********************************** Done
Summary
In this example, we have learn that how easily we can configure RSS and Atom feed into our spring boot project. Also we have seen how we can consume those from Java code. That’s all about today’s topic. I am attaching the full eclipse project here for your reference.
Please add your feedback in the comments section.
Happy Learning !!
Comments