Project Lombok is a very handy tool for removing the boilerplate code from the application. Lombok can also be used to configure logging in spring boot applications and thus remove the boilerplate code for getting the logger instance.
1. Setting Up Lombok with Spring Boot
Before using Lombok annotations, we must include lombok dependency in the Spring boot application. We may be required to enable the annotation processing in the IDEs such as installing Lombok into eclipse. IntelliJ automatically detects and configures lombok for us.
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
If we are creating a new project then we can choose Lombok in Spring Initializr page itself.
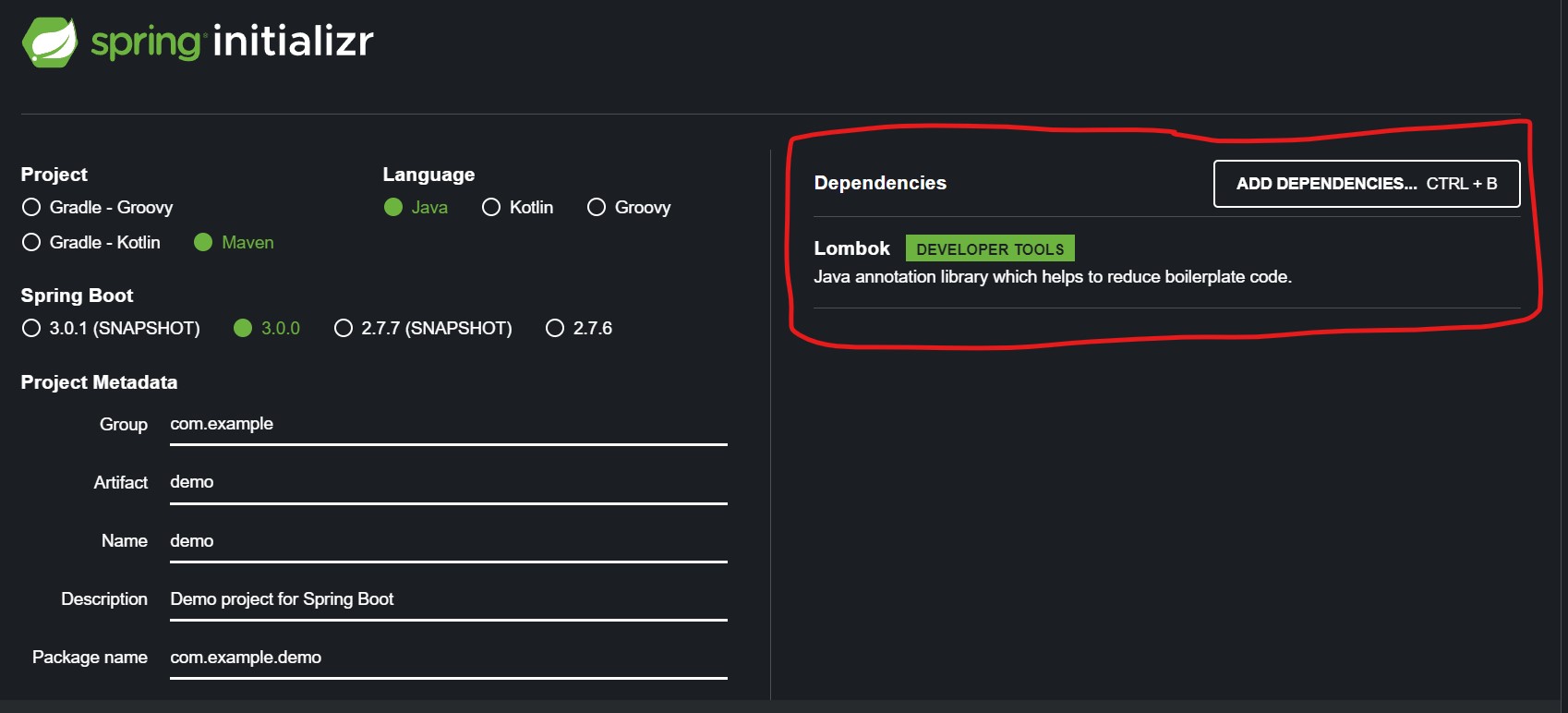
2. @Log, @Log4j2 and @Slf4j Annotations
Lombok supports the following annotations for logging statements in a spring boot application. These annotations let Lombok generate a logger field in the runtime. The logger name is always log
and the field’s type depends on which logger you have selected.
@CommonsLog – Creates the logger that logs using the Apache Commons Log API. The internally generated logger is:
private static final org.apache.commons.logging.Log log =
org.apache.commons.logging.LogFactory.getLog(MyClass.class);
@Flogger – Uses Google’s FluentLogger API for logging. The internally generated logger is:
private static final com.google.common.flogger.FluentLogger log =
com.google.common.flogger.FluentLogger.forEnclosingClass();
@Log – Uses Java Util Logging API for logging. The internally generated logger is:
private static final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MyClass.class.getName());
@Log4j2 – Uses Log4j2 API for logging. The internally generated logger is:
private static final org.apache.logging.log4j.Logger log =
org.apache.logging.log4j.LogManager.getLogger(MyClass.class);
@Slf4j – Uses SLF4j’s abstraction API and the logger library available on runtime for logging. The internally generated logger is:
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(MyClass.class);
To use any logger in a class, annotate the class with one of the above annotations and use the log for logging statements.
@Slf4j
public class Main {
public static void main(String... args) {
log.info("App is started!");
}
}
3. Using Log4j2 with Lombok
As we know spring boot uses logback as the default logging provider. To use log4j2, exclude logback from the classpath and include log4j2.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-log4j2</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
</dependencies>
As a best practice, we can use either @Slf4j
(recommended) or @Log4j2
as underlying logging implementation.
Happy Learning !!
Comments